Netty是基于流的消息傳遞機制。Netty框架中,所有消息的傳輸都依賴于ByteBuf接口,ByteBuf是Netty NIO框架中的緩沖區(qū)。ByteBuf接口可以理解為一般的Byte數(shù)組,不過Netty對Byte進行了封裝,增加了一些實用的方法。
ChannelBuf接口
package io.netty.buffer;
public interface ChannelBuf {
ChannelBufType type();
boolean isPooled();
}
ByteBuf接口
package io.netty.buffer;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.nio.channels.GatheringByteChannel;
import java.nio.channels.ScatteringByteChannel;
import java.nio.charset.Charset;
import java.nio.charset.UnsupportedCharsetException;
public interface ByteBuf extends ChannelBuf, Comparable<ByteBuf> {
int capacity();
ByteBuf capacity(int newCapacity);
int maxCapacity();
ByteOrder order();
ByteBuf order(ByteOrder endianness);
boolean isDirect();
int readerIndex();
ByteBuf readerIndex(int readerIndex);
int writerIndex();
ByteBuf writerIndex(int writerIndex);
ByteBuf setIndex(int readerIndex, int writerIndex);
int readableBytes();
int writableBytes();
boolean readable();
boolean writable();
ByteBuf clear();
ByteBuf markReaderIndex();
ByteBuf resetReaderIndex();
ByteBuf markWriterIndex();
ByteBuf resetWriterIndex();
ByteBuf discardReadBytes();
ByteBuf ensureWritableBytes(int minWritableBytes);
int ensureWritableBytes(int minWritableBytes, boolean force);
boolean getBoolean(int index);
byte getByte(int index);
short getUnsignedByte(int index);
short getShort(int index);
int getUnsignedShort(int index);
int getMedium(int index);
int getUnsignedMedium(int index);
int getInt(int index);
long getUnsignedInt(int index);
long getLong(int index);
char getChar(int index);
float getFloat(int index);
double getDouble(int index);
ByteBuf getBytes(int index, ByteBuf dst);
ByteBuf getBytes(int index, ByteBuf dst, int length);
ByteBuf getBytes(int index, ByteBuf dst, int dstIndex, int length);
ByteBuf getBytes(int index, byte[] dst);
ByteBuf getBytes(int index, byte[] dst, int dstIndex, int length);
ByteBuf getBytes(int index, ByteBuffer dst);
ByteBuf getBytes(int index, OutputStream out, int length) throws IOException;
int getBytes(int index, GatheringByteChannel out, int length) throws IOException;
ByteBuf setBoolean(int index, boolean value);
ByteBuf setByte(int index, int value);
ByteBuf setShort(int index, int value);
ByteBuf setMedium(int index, int value);
ByteBuf setInt(int index, int value);
ByteBuf setLong(int index, long value);
ByteBuf setChar(int index, int value);
ByteBuf setFloat(int index, float value);
ByteBuf setDouble(int index, double value);
ByteBuf setBytes(int index, ByteBuf src);
ByteBuf setBytes(int index, ByteBuf src, int length);
ByteBuf setBytes(int index, ByteBuf src, int srcIndex, int length);
ByteBuf setBytes(int index, byte[] src);
ByteBuf setBytes(int index, byte[] src, int srcIndex, int length);
ByteBuf setBytes(int index, ByteBuffer src);
int setBytes(int index, InputStream in, int length) throws IOException;
int setBytes(int index, ScatteringByteChannel in, int length) throws IOException;
ByteBuf setZero(int index, int length);
boolean readBoolean();
byte readByte();
short readUnsignedByte();
short readShort();
int readUnsignedShort();
int readMedium();
int readUnsignedMedium();
int readInt();
long readUnsignedInt();
long readLong();
char readChar();
float readFloat();
double readDouble();
ByteBuf readBytes(int length);
ByteBuf readSlice(int length);
ByteBuf readBytes(ByteBuf dst);
ByteBuf readBytes(ByteBuf dst, int length);
ByteBuf readBytes(ByteBuf dst, int dstIndex, int length);
ByteBuf readBytes(byte[] dst);
ByteBuf readBytes(byte[] dst, int dstIndex, int length);
ByteBuf readBytes(ByteBuffer dst);
ByteBuf readBytes(OutputStream out, int length) throws IOException;
int readBytes(GatheringByteChannel out, int length) throws IOException;
ByteBuf skipBytes(int length);
ByteBuf writeBoolean(boolean value);
ByteBuf writeByte(int value);
ByteBuf writeShort(int value);
ByteBuf writeMedium(int value);
ByteBuf writeInt(int value);
ByteBuf writeLong(long value);
ByteBuf writeChar(int value);
ByteBuf writeFloat(float value);
ByteBuf writeDouble(double value);
ByteBuf writeBytes(ByteBuf src);
ByteBuf writeBytes(ByteBuf src, int length);
ByteBuf writeBytes(ByteBuf src, int srcIndex, int length);
ByteBuf writeBytes(byte[] src);
ByteBuf writeBytes(byte[] src, int srcIndex, int length);
ByteBuf writeBytes(ByteBuffer src);
int writeBytes(InputStream in, int length) throws IOException;
int writeBytes(ScatteringByteChannel in, int length) throws IOException;
ByteBuf writeZero(int length);
int indexOf(int fromIndex, int toIndex, byte value);
int indexOf(int fromIndex, int toIndex, ByteBufIndexFinder indexFinder);
int bytesBefore(byte value);
int bytesBefore(ByteBufIndexFinder indexFinder);
int bytesBefore(int length, byte value);
int bytesBefore(int length, ByteBufIndexFinder indexFinder);
int bytesBefore(int index, int length, byte value);
int bytesBefore(int index, int length, ByteBufIndexFinder indexFinder);
ByteBuf copy();
ByteBuf copy(int index, int length);
ByteBuf slice();
ByteBuf slice(int index, int length);
ByteBuf duplicate();
boolean hasNioBuffer();
ByteBuffer nioBuffer();
ByteBuffer nioBuffer(int index, int length);
boolean hasNioBuffers();
ByteBuffer[] nioBuffers();
ByteBuffer[] nioBuffers(int offset, int length);
boolean hasArray();
byte[] array();
int arrayOffset();
String toString(Charset charset);
String toString(int index, int length, Charset charset);
@Override
int hashCode();
@Override
boolean equals(Object obj);
@Override
int compareTo(ByteBuf buffer);
@Override
String toString();
Unsafe unsafe();
interface Unsafe {
ByteBuffer nioBuffer();
ByteBuffer[] nioBuffers();
ByteBuf newBuffer(int initialCapacity);
void discardSomeReadBytes();
void acquire();
void release();
}
}

ByteBuf Index
ByteBuf通過兩個指針來協(xié)助I/O的讀寫操作,讀操作的readIndex和寫操作的writeIndex
readerIndex和writerIndex都是一開始都是0,隨著數(shù)據(jù)的寫入writerIndex會增加,讀取數(shù)據(jù)會使readerIndex增加,但是他不會超過writerIndx,在讀取之后,0-readerIndex的就被視為discard的.調(diào)用discardReadBytes方法,可以釋放這部分空間,他的作用類似ByeBuffer的compact方法;
讀和寫的時候Index是分開的,因此也就沒必要再每次讀完以后調(diào)用flip方法,另外還有indexOf、bytesBefore等一些方便的方法;
ByteBuf的幾個重要方法
discardReadBytes()
丟棄已讀的內(nèi)容。其執(zhí)行過程如下:
調(diào)用discardReadBytes()之前:
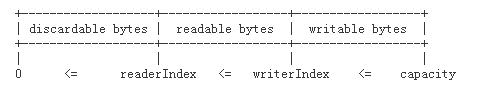
調(diào)用 discardReadBytes()方法后
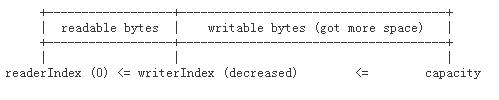
clear()
丟棄所有的數(shù)據(jù),并將readerIndex和writerIndex重置為0。
調(diào)用clear()之前
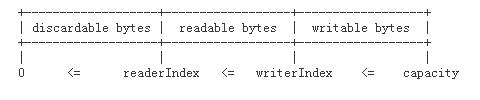
調(diào)用clear()之后
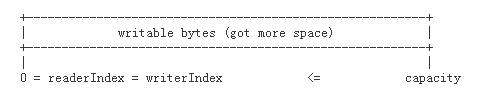
備注:因為筆者開始寫Netty源碼分析的時候,Netty 4.0還是處于Alpha階段,之后的API可能還會有改動,筆者將會及時更改。使用開源已經(jīng)有好幾年的時間了,一直沒有時間和精力來具體研究某個開源項目的具體實現(xiàn),這次是第一次寫開源項目的源碼分析,如果文中有錯誤的地方,歡迎讀者可以留言指出。對于轉(zhuǎn)載的讀者,請注明文章的出處。
希望和廣大的開發(fā)者/開源愛好者進行交流,歡迎大家的留言和討論。
-----------------------------------------------------
Silence, the way to avoid many problems;
Smile, the way to solve many problems;