1 JmxMBeanServer:
2 public Object getAttribute(ObjectName name, String attribute)
3 throws MBeanException, AttributeNotFoundException,
4 InstanceNotFoundException, ReflectionException {
5 //mbsInterceptor類型為DefaultMBeanServerInterceptor,也實現(xiàn)了MBeanServer接口,
6 //JmxMBeanServer實現(xiàn)的MBeanServer接口的方法,基本都是由mbs代理執(zhí)行的。
7 return mbsInterceptor.getAttribute(cloneObjectName(name), attribute);
8 }
9 10 DefaultMBeanServerInterceptor:
11 public Object getAttribute(ObjectName name, String attribute)
12 throws MBeanException, AttributeNotFoundException,
13 InstanceNotFoundException, ReflectionException {
14 
15 //通過ObjectName(key)從Repository中得到注冊過的MBean
16 final DynamicMBean instance = getMBean(name);
17 
18 //得到Attribute的值
19 return instance.getAttribute(attribute);
20 }
21 StandardMBeanSupport:
22 public final Object gtAttribute(String attribute)
23 throws AttributeNotFoundException,
24 MBeanException,
25 ReflectionException {
26 return perInterface.getAttribute(resource, attribute, getCookie());
27 }
28 PerInterface:
29 Object getAttribute(Object resource, String attribute, Object cookie)
30 throws AttributeNotFoundException,
31 MBeanException,
32 ReflectionException {
33 //得到Attribute屬性的方法,也就是MBean接口中定義的getXXX()方法
34 final M cm = getters.get(attribute);
35 if (cm ==
null) {
36 final String msg;
37 if (setters.containsKey(attribute))
38 msg = "Write-only attribute: " + attribute;
39 else40 msg = "No such attribute: " + attribute;
41 throw new AttributeNotFoundException(msg);
42 }
43 //在調(diào)用進去的話,就是通過反射調(diào)用cm方法,得到屬性的值
44 return introspector.invokeM(cm, resource, (Object[])
null, cookie);
45 }
MBeanInfo、MBeanAttributeInfo、MBeanConstructorInfo、MBeanNotificationInfo、MBeanOperationInfo:
這些類構(gòu)成了MBean的所有信息。JMX利用Introspection機制分析MBean的數(shù)據(jù),得到此MBean的元數(shù)據(jù)(i.e. 描述一個方法、屬性的名稱、類型、返回值等)。
MBeanAttributeInfo用于存放屬性相關(guān)的元數(shù)據(jù),MBeanConstructorInfo用于存放跟構(gòu)造器相關(guān)的元數(shù)據(jù),MBeanOperationInfo用于存放操作相關(guān)的元數(shù)據(jù),MBeanNotificationInfo用于存放JMX消息(事件)相關(guān)的元數(shù)據(jù)。MBeanInfo就是存放所有的這些元數(shù)新,JMX管理系統(tǒng)如果想知道一個MBean能管理的屬性或者能進行什么用的操作,那么都可以從MBeanInfo中獲得信息。
圖:MBeanInfo構(gòu)成
NotificationBroadcasterSupport、NotificationListener、NotificationFilter、NotificationBroadcaster:
這些類就是提供MBean的消息機制。給予一個MBean發(fā)送消息,過濾消息、監(jiān)聽消息,執(zhí)行消息等。一個MBean需要消息功能的話,就需要實現(xiàn)以后這些類。
圖:消息機制的結(jié)構(gòu)
Introspector、MBeanIntrospector:
JMX用這兩個類進行內(nèi)省,即通過他們能分析MBean的所有屬性、方法,然后進行封裝、轉(zhuǎn)化、存儲等,轉(zhuǎn)化成我們需要的數(shù)據(jù)結(jié)構(gòu)
MBeanRegistration :
用于一個MBean在注冊前后,或者注銷前后,做一些邏輯操作
圖:MBeanRegistration結(jié)構(gòu)圖 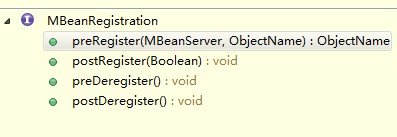
Dynamic、MBeanSupport、StandardMBeanSupport、MXBeanSupport:
即使MBean是標準形式的,但是JMX實現(xiàn)中,還是會生成一個動態(tài)的MBean,即StandardMBeanSupport,來封裝標準MBean。
圖:Dynamic、MBeanSupport、StandardMBeanSupport、MXBeanSupport關(guān)系圖(列出部分屬性、接口)
總結(jié):標準MBean按照一定編程規(guī)則(i.e. getXXX(),setXXX()),把需要管理的標準MBean的屬性和操作,加入到接口的方法中,然后標準MBean實現(xiàn)這個接口,這樣當標準MBean注冊到MBeanServer中后,MBeanServer就可以管理此MBean了。標準MBean在于想要新增一個管理的屬性或操作,都要先在接口中先新增一個方法,然后實現(xiàn)。
DynamicMBeanJMX管理的MBean除了標準MBean外,還可以是DynamicMBean。只要我們實現(xiàn)此接口,就可以被JMX Server管理。
例子:
1 package test.jmx;
2
3 import java.lang.reflect.Method;
4
5 import javax.management.Attribute;
6 import javax.management.AttributeList;
7 import javax.management.AttributeNotFoundException;
8 import javax.management.DynamicMBean;
9 import javax.management.InvalidAttributeValueException;
10 import javax.management.MBeanAttributeInfo;
11 import javax.management.MBeanException;
12 import javax.management.MBeanInfo;
13 import javax.management.MBeanOperationInfo;
14 import javax.management.ReflectionException;
15
16 public class HelloWorldDynamic implements DynamicMBean {
17 public String hello;
18
19
20 public HelloWorldDynamic() {
21 this.hello = "Hello World! I am a Dynamic MBean";
22 }
23
24 public HelloWorldDynamic(String hello) {
25 this.hello = hello;
26 }
27
28 public String getHello() {
29 return hello;
30 }
31
32 public Object getInstance() {
33 return new Object();
34 }
35
36 public void setHello(String hello) {
37 this.hello = hello;
38 }
39
40 @Override
41 public Object getAttribute(String attribute)
42 throws AttributeNotFoundException, MBeanException,
43 ReflectionException {
44 //設置getAttribute的執(zhí)行邏輯
45 if("getInstance".equals(attribute)){
46 return getInstance();
47 }
48
49 return null;
50 }
51
52 @Override
53 public AttributeList getAttributes(String[] attributes) {
54 // TODO Auto-generated method stub
55 return null;
56 }
57
58 MBeanInfo info = null;
59 @Override
60 public MBeanInfo getMBeanInfo() {
61 try {
62 Class cls = this.getClass();
63 // 用反射獲得 "getHello" 屬性的讀方法
64 //DynamicMBean中,
65 Method readMethod = cls.getMethod("getHello", new Class[0]);
66 MBeanAttributeInfo attribute = new MBeanAttributeInfo("gh",
67 " the first attribute ", readMethod, null);
68 //執(zhí)行java類的method需要的一些元數(shù)據(jù),由MBeanOperationInfo提供
69 MBeanOperationInfo operation = new MBeanOperationInfo(
70 " the first operation ", cls.getMethod("getInstance", null));
71 info = new MBeanInfo(cls.getName(), " this is a dynamic MBean ",
72 new MBeanAttributeInfo[] { attribute }, null,
73 new MBeanOperationInfo[] { operation }, null);
74 } catch (Exception e) {
75 System.out.println(e);
76 }
77 return info;
78 }
79
80 @Override
81 public Object invoke(String actionName, Object[] params, String[] signature)
82 throws MBeanException, ReflectionException {
83 System.out.println(" the HelloWorldDynamic's method invoke ");
84 return null;
85 }
86
87 @Override
88 public void setAttribute(Attribute attribute)
89 throws AttributeNotFoundException, InvalidAttributeValueException,
90 MBeanException, ReflectionException {
91
92 }
93
94 @Override
95 public AttributeList setAttributes(AttributeList attributes) {
96 return null;
97 }
98 }
99
測試此MBean請看JmxTest類中的test2DynamicMBean()。
當實現(xiàn)一個DynamicMBean,我們需要寫的代碼量是非常多的,MBeanInfo的信息,需要自己編碼,而且對于MBeanServer操作MBean的方法,也得自己重載實現(xiàn)。在動態(tài)MBean中,MBeanInfo里面的信息,主要用來展示的,具體的對DynamicMBean的操作是自己實現(xiàn)的。DynamicMBean的優(yōu)點是對于邏輯控制是可以很靈活的。而不像標準MBean一樣,所有的操作或?qū)傩孕枰贛Bean接口中定義。在jdk中JMX實現(xiàn)DynamicMBean的流程是非常簡單的,Jmx server對于DynamicMBean的操作也是非常簡單的,相對于標準MBean,注冊的時候少了內(nèi)省的步驟;其他的操作跟標準MBean一樣,只是對于getAttribute(...),setAttribute(...),invoke(...)等一些的操作都是需要自己來實現(xiàn)的。
ModelMBean 然而,普通的動態(tài) Bean通常缺乏一些管理系統(tǒng)所需要的支持:比如持久化MBean的狀態(tài)、日志記錄、緩存等等。如果讓用戶去一一實現(xiàn)這些功能確實是件枯燥無聊的工作。為了減輕用戶的負擔,JMX提供商都會提供不同的 ModelBean實現(xiàn)。其中有一個接口是 Java 規(guī)范中規(guī)定所有廠商必須實現(xiàn)的:
javax.management.modelmbean.RequiredModelBean
。通過配置Descriptor信息,我們可以定制這個ModelBean,指定哪些 MBean狀態(tài)需要記入日志、如何記錄以及是否緩存某些屬性、緩存多久等等。對于Descriptor,在MBean中相當于附帶的一些信息,這些信息在MBean實現(xiàn)中可以作為一種策略,以此增強MBean的功能。動態(tài)MBean以及標準MBean的MBeanInfo都已經(jīng)包括了Descriptor,但是在邏輯實現(xiàn)中沒用到此對象。在ModelMBean中,Descriptor作用非常大,持久化、日志、緩存等的策略等相關(guān)信息都是在Descriptor中定義的。開發(fā)人員可以增加相關(guān)屬性到Descriptor中,來對應用功能進行擴展
這里,我們以 RequiredModelBean 為例討論 ModelBean。
例子: 1 package test.jmx;
2 3 import java.lang.reflect.Constructor;
4 5 import javax.management.Descriptor;
6 import javax.management.InstanceNotFoundException;
7 import javax.management.MBeanException;
8 import javax.management.MBeanOperationInfo;
9 import javax.management.MBeanParameterInfo;
10 import javax.management.RuntimeOperationsException;
11 import javax.management.modelmbean.DescriptorSupport;
12 import javax.management.modelmbean.InvalidTargetObjectTypeException;
13 import javax.management.modelmbean.ModelMBeanAttributeInfo;
14 import javax.management.modelmbean.ModelMBeanConstructorInfo;
15 import javax.management.modelmbean.ModelMBeanInfo;
16 import javax.management.modelmbean.ModelMBeanInfoSupport;
17 import javax.management.modelmbean.ModelMBeanOperationInfo;
18 import javax.management.modelmbean.RequiredModelMBean;
19 20 public class HelloWorldModelMBean
extends RequiredModelMBean {
21 22 public HelloWorldModelMBean()
throws Exception{}
23 24 public static RequiredModelMBean createModelBean()
25 throws RuntimeOperationsException, MBeanException,
26 InstanceNotFoundException, InvalidTargetObjectTypeException {
27 // 模型MBean信息
28 ModelMBeanInfo info = buildModelMBeanInfo();
29 // 模型MBean
30 RequiredModelMBean modelMBean =
new RequiredModelMBean(info);
31 //目前只支持ObjectReference,將來可能會支持ObjectReference", "Handle", "IOR", "EJBHandle",
32 // or "RMIReference,
33 //RMIReference從名字上可以看出,如果支持的話,那么以后就可以支持遠程MBean引用
34 modelMBean.setManagedResource(
new HelloWorld(), "ObjectReference");
35 return modelMBean;
36 }
37 38 protected static ModelMBeanInfo buildModelMBeanInfo()
throws RuntimeOperationsException, MBeanException {
39 // --
40 // attributes
41 // ------------------------------------------------------------------
42 ModelMBeanAttributeInfo[] attributes =
new ModelMBeanAttributeInfo[1];
43 44 // 設置屬性
45 Descriptor nameDesc =
new DescriptorSupport();
46 nameDesc.setField("name", "hello");
47 nameDesc.setField("value", "----dfdf---");
48 nameDesc.setField("displayName", "myname");
49 nameDesc.setField("setMethod", "setHello");
50 nameDesc.setField("getMethod", "getHello");
51 nameDesc.setField("descriptorType", "attribute");
52 attributes[0] =
new ModelMBeanAttributeInfo("hello", "java.lang.String",
53 "name say hello to",
true,
true,
false, nameDesc);
54 55 // --
56 // operations
57 // -------------------------------------------------------------------
58 ModelMBeanOperationInfo[] operations =
new ModelMBeanOperationInfo[2];
59 String className = HelloWorld.
class.getName();
60 61 // getName method
62 Descriptor getDesc =
new DescriptorSupport(
new String[] {
63 "name=getHello", "descriptorType=operation",
64 "class=" + className, "role=operation" });
65 operations[0] =
new ModelMBeanOperationInfo("getHello", "get hello

",
66 null,
null, MBeanOperationInfo.ACTION, getDesc);
67 68 Descriptor setDesc =
new DescriptorSupport(
new String[] {
69 "name=setHello", "descriptorType=operation",
70 "class=" + className, "role=operation" });
71 operations[1] =
new ModelMBeanOperationInfo("setHello", "set hello

",
72 new MBeanParameterInfo[]{
new MBeanParameterInfo("a","java.lang.String"," a method's arg ")},
73 null, MBeanOperationInfo.ACTION, setDesc);
74 75 // constructors
76 ModelMBeanConstructorInfo[] constructors =
new ModelMBeanConstructorInfo[1];
77 Constructor<?>[] ctors = HelloWorld.
class.getConstructors();
78 79 80 constructors[0] =
new ModelMBeanConstructorInfo("default constructor",
81 ctors[0],
null);
82 83 // ModelMBeanInfo
84 ModelMBeanInfo mmbeanInfo =
new ModelMBeanInfoSupport(className,
85 "Simple implementation of model bean.", attributes,
null,
86 operations
/*null*/,
null,
null);
87 88 //設置一個Descriptor策略,這樣RequiredModelMBean改變 Attribute值得時候會記錄日志
89 //當然RequiredModelMBean還需要addAttributeChangeNotificationListener,注冊一個監(jiān)聽器
90 Descriptor globalDescriptor =
new DescriptorSupport(
new String[]{
91 "name=HelloWorldModelMBean","displayName=globaldescriptor",
92 "descriptorType=mbean","log=T","logfile=hello.log"
93 });
94 mmbeanInfo.setMBeanDescriptor(globalDescriptor);
95 96 return mmbeanInfo;
97 }
98 99 }
100 使用ModelMBean中,有兩步很重要,第一步設置動態(tài)MBean元數(shù)據(jù):setModelMBeanInfo(...),MBeanServer會利用這些元數(shù)據(jù)管理MBean。第二步設置ModelMBean需要管理的對象:setManagerdResourece(...),第一步的元數(shù)據(jù)其實也就是被管理對象的元數(shù)據(jù)。這二步都是可以在運行時候動態(tài)的配置的,對于ModelMBeanInfo和Resource等相關(guān)信息可以在xml文件中進行配置。所以對于ModelMBean的實現(xiàn),可以很好的利用xml等工具。
測試此MBean請看JmxTest類中的test3RequiredModelMBean()方法。
代碼:RequiredModelMBean.setAttribute(...)的執(zhí)行分析 1 JmxMBeanServer:
2 public void setAttribute(ObjectName name, Attribute attribute)
3 throws InstanceNotFoundException, AttributeNotFoundException,
4 InvalidAttributeValueException, MBeanException,
5 ReflectionException {
6 7 mbsInterceptor.setAttribute(cloneObjectName(name),
8 cloneAttribute(attribute));
9 }
10 11 DefaultMBeanServerInterceptor:
12 public void setAttribute(ObjectName name, Attribute attribute)
13 throws InstanceNotFoundException, AttributeNotFoundException,
14 InvalidAttributeValueException, MBeanException,
15 ReflectionException {
16 
.
17 //得到動態(tài)MBean
18 DynamicMBean instance = getMBean(name);
19 instance.setAttribute(attribute);
20 
.
21 }
22 23 RequiredModelMBean:
24 public void setAttribute(Attribute attribute)
25 throws AttributeNotFoundException, InvalidAttributeValueException,
26 MBeanException, ReflectionException
27 
.
28 //modelMBeanInfo就是最開始創(chuàng)建的信息,得到一個AttributeInfo
29 ModelMBeanAttributeInfo attrInfo =
30 modelMBeanInfo.getAttribute(attrName);
31 
.
32 Descriptor mmbDesc = modelMBeanInfo.getMBeanDescriptor();
33 Descriptor attrDescr = attrInfo.getDescriptor();
34 
.
35 //得到set方法名
36 String attrSetMethod = (String)
37 (attrDescr.getFieldValue("setMethod"));
38 //得到get方法名
39 String attrGetMethod = (String)
40 (attrDescr.getFieldValue("getMethod"));
41 
.
42 //更具必要參數(shù),執(zhí)行set方法。改變被管理資源的值
43 invoke(attrSetMethod,
44 (
new Object[] {attrValue}),
45 (
new String[] {attrType}) );
46 
.
47 //發(fā)出Attribute改變的事件
48 sendAttributeChangeNotification(oldAttr,attribute);
49 
.
50 }
51 public void sendAttributeChangeNotification(AttributeChangeNotification
52 ntfyObj)
53 throws MBeanException, RuntimeOperationsException {
54 
.
55 // log notification if specified in descriptor
56 Descriptor ntfyDesc =
57 modelMBeanInfo.getDescriptor(ntfyObj.getType(),"notification");
58 //這個就是在例子中設置的globalDescriptor
59 Descriptor mmbDesc = modelMBeanInfo.getMBeanDescriptor();
60 
.
61 if (mmbDesc !=
null) {
62 //這些值都是我們設置在globalDescriptor的策略,也就是具體 //需要JMX的實現(xiàn)這需要各自實現(xiàn)的策略
63 logging = (String) mmbDesc.getFieldValue("log");
64 if ((logging !=
null) &&
65 ( logging.equalsIgnoreCase("t") ||
66 logging.equalsIgnoreCase("true") )) {
67 logfile = (String) mmbDesc.getFieldValue("logfile");
68 69 if (logfile !=
null) {
70 try {
71 //把相關(guān)信息寫入日志
72 writeToLog(logfile,"LogMsg: " +
73 ((
new Date(ntfyObj.getTimeStamp())).toString())+
74 " " + ntfyObj.getType() + " " +
75 ntfyObj.getMessage() +
76 " Name = " + ntfyObj.getAttributeName() +
77 " Old value = " + oldv +
78 " New value = " + newv);
79 }
80 
81 }
82 在ModelMBean中一些重要的類:ModelMBean:
實現(xiàn)了DynamicMBean,說明了ModelMBean也是動態(tài)MBean的一類,PersistentMBean持久化功能接口,還實現(xiàn)了消息機制。
圖:ModelMBean的結(jié)構(gòu)圖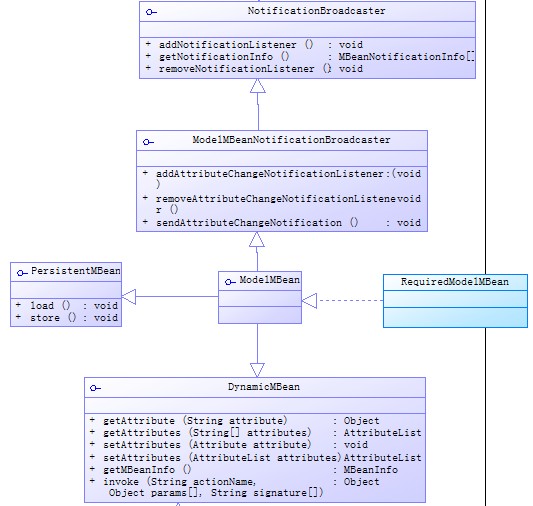
ModelMBeanInfo、ModelMBeanAttributeInfo、ModelMBeanConstructorInfo、ModelMBeanNotificationInfo、ModelMBeanOperationInfo:
這些類跟DynamicMBean里面介紹的類很相似,這里的ModelXXX都是XXX的子類。而且構(gòu)成也跟他們的父類是一樣的,子類只是擴展了一些信息。
RequiredModelMBean:
RequiredModelMBean實現(xiàn)了ModelMBean其實實現(xiàn)了DynamicMBean,其實它也是一個動態(tài)的MBean,規(guī)范中說明對于使用ModelMBean,第三方供應商都必須實現(xiàn)RequiredMoelMBean。
參考:
http://www.ibm.com/developerworks/cn/java/j-lo-jse63/index.html(
Java SE 6 新特性: JMX 與系統(tǒng)管理)