public class GZIPcompress {
public static void main(String[] args) throws IOException {
BufferedReader in = new BufferedReader(
new FileReader("test.txt"));
BufferedOutputStream out = new BufferedOutputStream(
new GZIPOutputStream(new FileOutputStream("test.gz")));
System.out.println("Writing file
");
int c;
while((c = in.read())!=-1){
out.write(c);
}
in.close();
out.close();
System.out.println("Reading file
");
BufferedReader in2 = new BufferedReader(
new InputStreamReader(new GZIPInputStream(new FileInputStream("test.gz"))));
String s;
while((s=in2.readLine())!=null){
System.out.println(s);
}
}
} The use of the compression classes is straightforward; you simply wrap your output stream in a GZIPOutputStream or ZipOutputStream, and your input stream in a GZIPInputStream or ZipInputStream. All else is ordinary I/O reading and writing. This is an example of mixing the char-oriented streams with the byte-oriented streams; in uses the Reader classes, whereas GZIPOutputStream’s constructor can accept only an OutputStream object, not a Writer object. When the file is opened, the GZIPInputStream is converted to a Reader.
Multifile storage with Zip
it shows the use of the Checksum classes to calculate and verify the checksum for the file. There are two Checksum types: Adler32 (which is faster) and CRC32 (which is slower but slightly more accurate).
/**
* Uses ZIP compression to compress any number of files given on the command line.
* @author WPeng
*
* @since 2012-11-8
*/
public class ZipCompress {
public static void main(String[] args) throws IOException{
FileOutputStream f = new FileOutputStream("test.zip");
CheckedOutputStream csum = new CheckedOutputStream(f, new Adler32());
ZipOutputStream zos = new ZipOutputStream(csum);
BufferedOutputStream out = new BufferedOutputStream(zos);
zos.setComment("A test of Java Zipping");
// No corresponding getComment(), though
for(String arg : args){
System.out.println("Wrting file " + arg);
BufferedReader in = new BufferedReader(new FileReader(arg));
zos.putNextEntry(new ZipEntry(arg));
int c;
while((c = in.read())!=-1)
out.write(c);
in.close();
out.flush();
}
// Checksum valid only after the file has been closed!
System.out.println("Checksum: " + csum.getChecksum().getValue());
// Now extract the files:
System.out.println("Reading file");
FileInputStream fin = new FileInputStream("test.zip");
CheckedInputStream csumi = new CheckedInputStream(fin, new Adler32());
ZipInputStream in2 = new ZipInputStream(csumi);
BufferedInputStream bis = new BufferedInputStream(in2);
ZipEntry ze;
while((ze = in2.getNextEntry()) != null){
System.out.println("Reading file " + ze);
int x;
while((x=bis.read()) != -1)
System.out.println(x);
}
if(args.length==1){
System.out.println("Checksum: " + csumi.getChecksum().getValue());
}
bis.close();
// Alternative way to open and read Zip files:
ZipFile zf = new ZipFile("test.zip");
Enumeration e = zf.entries();
while(e.hasMoreElements()){
ZipEntry ze2 = (ZipEntry)e.nextElement();
System.out.println("File: "+ ze2);
//
and extract the data as before
}
}
} For each file to add to the archive, you must call putNextEntry( ) and pass it a ZipEntry object.
The ZipEntry object contains an extensive interface that allows you to get and set all the data available on that particular entry in your Zip file: name, compressed and uncompressed sizes, date, CRC checksum, extra field data, comment, compression method, and whether it’s a directory entry.
CheckedInputStream and CheckedOutputStream support both Adler32 and CRC32 checksums, the ZipEntry class supports only an interface for CRC
To extract files, ZipInputStream has a getNextEntry( ) method that returns the next ZipEntry if there is one
JAR files are cross-platform, so you don’t need to worry about platform issues. You can also include audio and image files as well as class files.
JAR files are particularly helpful when you deal with the Internet.
jar [options] destination [manifest] inputfile(s)
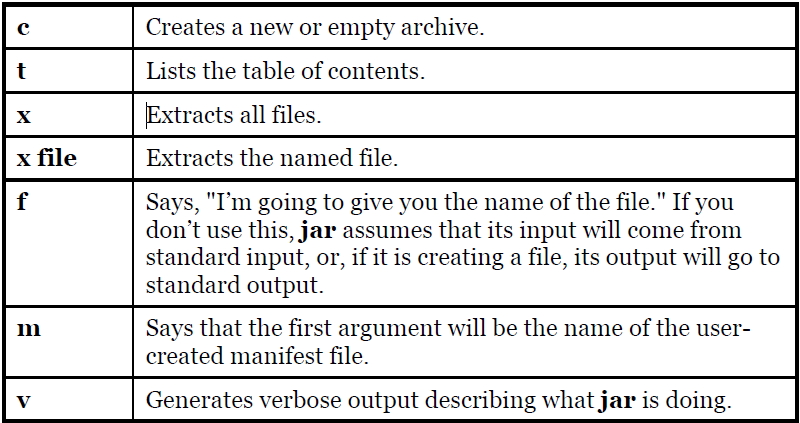

Here are some typical ways to invoke jar. The following command creates a JAR file called myJarFile.jar that contains all of the class files in the current directory, along with an automatically generated manifest file:
jar cf myJarFile.jar *.class
The next command is like the previous example, but it adds a user-created manifest file called myManifestFile.mf:
jar cmf myJarFile.jar myManifestFile.mf *.class
This produces a table of contents of the files in myJarFile.jar:
jar tf myJarFile.jar
This adds the "verbose" flag to give more detailed information about the files in myJarFile.jar:
jar tvf myJarFile.jar
Assuming audio, classes, and image are subdirectories, this combines all of the subdirectories into the file myApp.jar. The "verbose" flag is also included to give extra feedback while the jar program is working:
jar cvf myApp.jar audio classes image
a JAR file created on one platform will be transparently readable by the jar tool on any other platform (a problem that sometimes plagues Zip utilities).