Cobertura是一個基于jcoverage的免費Java工具,它能夠顯示哪一部分代碼被你的測試所覆蓋,并可生成HTML或XML報告. 本文將介紹如何在項目中使用cobertura來測量單元測試的代碼覆蓋情況.
首先創建一個Java項目,本文以Eclipse為例:
項目名: CodeCoverageTest
結構圖如下:
接下,創建一個Ant構建配置文件,幫我們實現自動化的編譯,單元測試以及cobertura來測量單元測試的代碼覆蓋情況。
Ant的 build.xml文件內容如下:
1 <?xml version="1.0" encoding="UTF-8"?>
2
3 <project name="cobertura.examples.basic" default="coverage" basedir=".">
4
5 <!-- 引用 build.properties文件 配置路徑信息-->
6 <property file="build.properties" />
7
8 <!-- 設置 cobertura路徑 -->
9 <path id="cobertura.classpath">
10 <fileset dir="${cobertura.dir}">
11 <include name="cobertura.jar" />
12 <include name="lib/**/*.jar" />
13 </fileset>
14 </path>
15
16 <!-- 配置 cobatura ant 擴展任務 -->
17 <taskdef classpathref="cobertura.classpath" resource="tasks.properties"/>
18
19 <target name="init">
20 <mkdir dir="${classes.dir}" />
21 <mkdir dir="${instrumented.dir}" />
22 <mkdir dir="${reports.xml.dir}" />
23 <mkdir dir="${reports.html.dir}" />
24 <mkdir dir="${coverage.xml.dir}" />
25 <mkdir dir="${coverage.html.dir}" />
26 </target>
27
28 <!-- 編譯源代碼 -->
29 <target name="compile" depends="init">
30 <javac srcdir="${src.dir}:${test.dir}:${src.conf.dir}:${test.conf.dir}" destdir="${classes.dir}" debug="yes">
31 <classpath refid="cobertura.classpath" />
32 </javac>
33 </target>
34
35 <target name="instrument" depends="init,compile">
36 <!--
37 Remove the coverage data file and any old instrumentation.
38 -->
39 <delete file="cobertura.ser"/>
40 <delete dir="${instrumented.dir}" />
41
42 <!--
43 Instrument the application classes, writing the
44 instrumented classes into ${build.instrumented.dir}.
45 -->
46 <cobertura-instrument todir="${instrumented.dir}">
47 <!--
48 The following line causes instrument to ignore any
49 source line containing a reference to log4j, for the
50 purposes of coverage reporting.
51 -->
52 <ignore regex="org.apache.log4j.*" />
53
54 <fileset dir="${classes.dir}">
55 <!--
56 Instrument all the application classes, but
57 don't instrument the test classes.
58 -->
59 <include name="**/*.class" />
60 <exclude name="**/*Test.class" />
61 </fileset>
62 </cobertura-instrument>
63 </target>
64
65 <!-- 單元測試 -->
66 <target name="test" depends="init,compile">
67 <junit fork="yes" dir="${basedir}" failureProperty="test.failed">
68 <!--
69 Note the classpath order: instrumented classes are before the
70 original (uninstrumented) classes. This is important.
71 -->
72 <classpath location="${instrumented.dir}" />
73 <classpath location="${classes.dir}" />
74
75 <!--
76 The instrumented classes reference classes used by the
77 Cobertura runtime, so Cobertura and its dependencies
78 must be on your classpath.
79 -->
80 <classpath refid="cobertura.classpath" />
81
82 <formatter type="xml" />
83 <test name="${testcase}" todir="${reports.xml.dir}" if="testcase" />
84 <batchtest todir="${reports.xml.dir}" unless="testcase">
85 <fileset dir="${test.dir}">
86 <include name="**/*Test.java" />
87 </fileset>
88 <fileset dir="${src.dir}">
89 <include name="**/*Test.java" />
90 </fileset>
91 </batchtest>
92 </junit>
93
94 <junitreport todir="${reports.xml.dir}">
95 <fileset dir="${reports.xml.dir}">
96 <include name="TEST-*.xml" />
97 </fileset>
98 <report format="frames" todir="${reports.html.dir}" />
99 </junitreport>
100 </target>
101
102 <target name="coverage-check">
103 <cobertura-check branchrate="34" totallinerate="100" />
104 </target>
105
106 <!-- 生成 coverage xml格式報告 -->
107 <target name="coverage-report">
108 <!--
109 Generate an XML file containing the coverage data using
110 the "srcdir" attribute.
111 -->
112 <cobertura-report srcdir="${src.dir}" destdir="${coverage.xml.dir}" format="xml" />
113 </target>
114
115 <!-- 生成 coverage html格式報告 -->
116 <target name="alternate-coverage-report">
117 <!--
118 Generate a series of HTML files containing the coverage
119 data in a user-readable form using nested source filesets.
120 -->
121 <cobertura-report destdir="${coverage.html.dir}">
122 <fileset dir="${src.dir}">
123 <include name="**/*.java"/>
124 </fileset>
125 </cobertura-report>
126 </target>
127
128 <target name="clean" description="Remove all files created by the build/test process.">
129 <delete dir="${classes.dir}" />
130 <delete dir="${instrumented.dir}" />
131 <delete dir="${reports.dir}" />
132 <delete file="cobertura.log" />
133 <delete file="cobertura.ser" />
134 </target>
135
136 <target name="coverage" depends="compile,instrument,test,coverage-report,alternate-coverage-report" description="Compile, instrument ourself, run the tests and generate JUnit and coverage reports."/>
137
138 </project>
139
build.properties文件
1 # The source code for the examples can be found in this directory
2 src.dir=../src/java
3 src.conf.dir=../src/conf
4 test.dir=../test/java
5 test.conf.dir=../src/conf
6
7 # The path to cobertura.jar
8 cobertura.dir=cobertura
9
10 # Classes generated by the javac compiler are deposited in this directory
11 classes.dir=../bin
12
13 # Instrumented classes are deposited into this directory
14 instrumented.dir=instrumented
15
16 # All reports go into this directory
17 reports.dir=reports
18
19 # Unit test reports from JUnit are deposited into this directory
20 reports.xml.dir=${reports.dir}/junit-xml
21 reports.html.dir=${reports.dir}/junit-html
22
23 # Coverage reports are deposited into these directories
24 coverage.xml.dir=${reports.dir}/cobertura-xml
25 coverage.html.dir=${reports.dir}/cobertura-html
26
編寫示例代碼
Hello.java
1 package com.xmatthew.practise.cobertura;
2
3 public class Hello {
4
5 public String birthday(String name) {
6 return "happy birthday to " + name;
7 }
8
9 public String christmas(String name) {
10 return "merry christmas " + name;
11 }
12
13 }
編寫測試Hello.java的測試類,進行單元測試
HelloTest.java
1 package com.xmatthew.practise.cobertura;
2
3 import junit.framework.Assert;
4 import junit.framework.TestCase;
5
6 public class HelloTest extends TestCase {
7
8 private Hello hello;
9
10 protected void setUp() throws Exception {
11 hello = new Hello();
12 }
13
14 protected void tearDown() throws Exception {
15 hello = null;
16 }
17
18 public void testBirthday() {
19 String expected = "happy birthday to matt";
20 Assert.assertEquals(expected, hello.birthday("matt"));
21 }
22
23 public void testChristmas() {
24 String expected = "merry christmas matt";
25 Assert.assertEquals(expected, hello.christmas("matt"));
26 }
27
28 }
29
接下來,我們運行 Cobertura來進行測試
運行 Cobertura,測試在Eclipse運行時,要在Ant插件的運行類庫環境中加入cobertura相關類庫。
查看運行結果報告:
JUnit報告
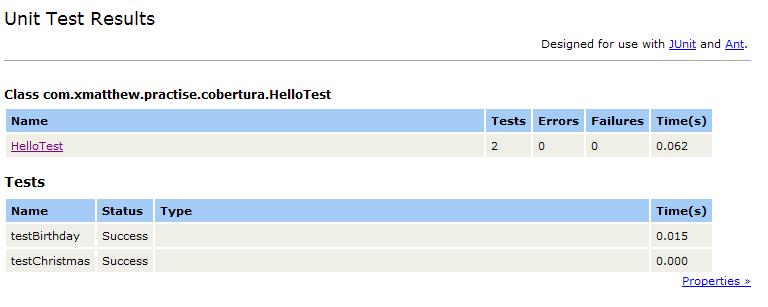
Cobertura報告
到目前已經完成整個項目的基于Ant進行編譯,測試以及生成Cobertura測試報告的開發IDE環境。這個項目框架足以應用日常的開發需求。
Cobertura官網地址:
http://cobertura.sourceforge.net/
Good Luck!
Yours Matthew!