應朋友要求,寫了一個小工具,主要就是實現下面的要求:
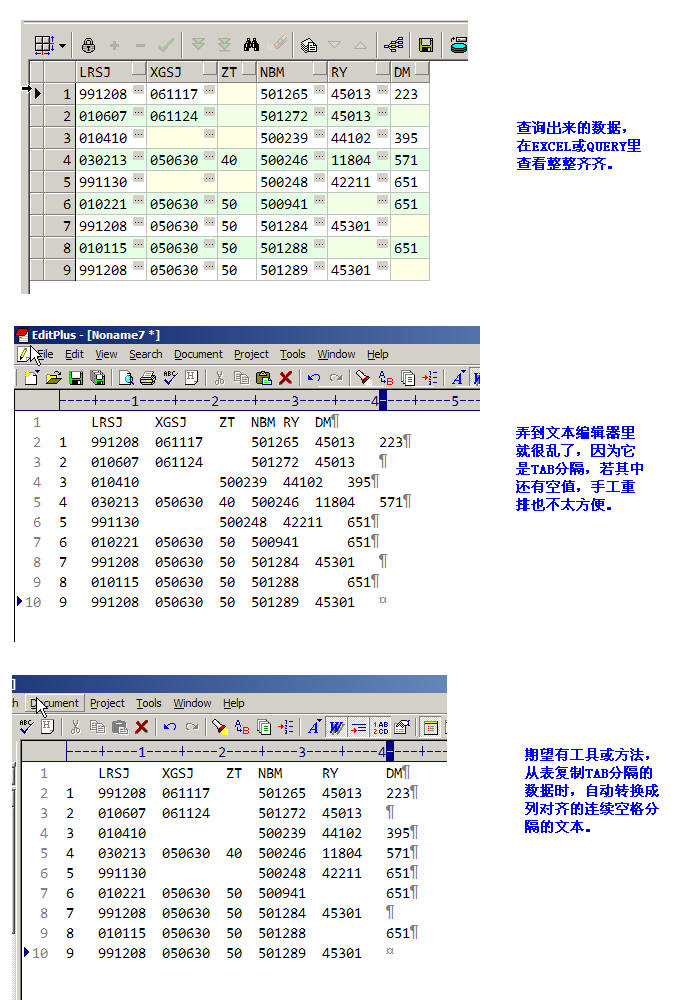
??1?import?java.util.LinkedList;
??2?
??3?import?org.eclipse.swt.SWT;
??4?import?org.eclipse.swt.events.KeyAdapter;
??5?import?org.eclipse.swt.events.KeyEvent;
??6?import?org.eclipse.swt.events.SelectionAdapter;
??7?import?org.eclipse.swt.events.SelectionEvent;
??8?import?org.eclipse.swt.layout.FormAttachment;
??9?import?org.eclipse.swt.layout.FormData;
?10?import?org.eclipse.swt.layout.FormLayout;
?11?import?org.eclipse.swt.widgets.Button;
?12?import?org.eclipse.swt.widgets.Display;
?13?import?org.eclipse.swt.widgets.Label;
?14?import?org.eclipse.swt.widgets.Shell;
?15?import?org.eclipse.swt.widgets.Text;
?16?
?17?public?class?FormatSqlResult?{
?18?
?19?????private?Text?text;
?20?
?21?????protected?Shell?shell;
?22?
?23?????/**
?24??????*?Launch?the?application
?25??????*?
?26??????*?@param?args
?27??????*/
?28?????public?static?void?main(String[]?args)?{
?29?????????try?{
?30?????????????FormatSqlResult?window?=?new?FormatSqlResult();
?31?????????????window.open();
?32?????????}?catch?(Exception?e)?{
?33?????????????e.printStackTrace();
?34?????????}
?35?????}
?36?
?37?????/**
?38??????*?Open?the?window
?39??????*/
?40?????public?void?open()?{
?41?????????final?Display?display?=?Display.getDefault();
?42?????????createContents();
?43?????????shell.open();
?44?????????shell.layout();
?45?????????while?(!shell.isDisposed())?{
?46?????????????if?(!display.readAndDispatch())
?47?????????????????display.sleep();
?48?????????}
?49?????}
?50?
?51?????/**
?52??????*?Create?contents?of?the?window
?53??????*/
?54?????protected?void?createContents()?{
?55?????????shell?=?new?Shell();
?56?????????shell.setLayout(new?FormLayout());
?57?????????shell.setSize(631,?414);
?58?????????shell.setText("FormatSqlResult");
?59?
?60?????????text?=?new?Text(shell,?SWT.V_SCROLL?|?SWT.MULTI?|?SWT.H_SCROLL?|?SWT.BORDER);
?61?????????text.addKeyListener(new?KeyAdapter()?{
?62?????????????public?void?keyPressed(KeyEvent?e)?{
?63?????????????????System.out.println(e.keyCode);
?64?????????????}
?65?????????});
?66?????????final?FormData?fd_text?=?new?FormData();
?67?????????fd_text.bottom?=?new?FormAttachment(100,?-34);
?68?????????fd_text.right?=?new?FormAttachment(100,?-5);
?69?????????fd_text.left?=?new?FormAttachment(0,?0);
?70?????????fd_text.top?=?new?FormAttachment(0,?0);
?71?????????text.setLayoutData(fd_text);
?72?????????final?Button?formatButton?=?new?Button(shell,?SWT.NONE);
?73?????????formatButton.addSelectionListener(new?SelectionAdapter()?{
?74?????????????public?void?widgetSelected(SelectionEvent?e)?{
?75?????????????????String?str?=?text.getText();
?76?????????????????if?(str?!=?null?&&?str.length()?>?0)?{
?77?????????????????????text.setText(getSpaceText(str.replaceAll("\r",?"")));
?78?????????????????????text.selectAll();
?79?????????????????}
?80?
?81?????????????}
?82?????????});
?83?????????final?FormData?fd_formatButton?=?new?FormData();
?84?????????fd_formatButton.left?=?new?FormAttachment(0,?286);
?85?????????fd_formatButton.right?=?new?FormAttachment(100,?-287);
?86?????????fd_formatButton.top?=?new?FormAttachment(100,?-26);
?87?????????fd_formatButton.bottom?=?new?FormAttachment(100,?-4);
?88?????????formatButton.setLayoutData(fd_formatButton);
?89?????????formatButton.setText("Format");
?90?
?91?????????final?Label?label?=?new?Label(shell,?SWT.NONE);
?92?????????final?FormData?fd_label?=?new?FormData();
?93?????????fd_label.top?=?new?FormAttachment(100,?-19);
?94?????????fd_label.left?=?new?FormAttachment(100,?-130);
?95?????????fd_label.bottom?=?new?FormAttachment(100,?-4);
?96?????????fd_label.right?=?new?FormAttachment(100,?-5);
?97?????????label.setLayoutData(fd_label);
?98?????????label.setText("版權所有:交口稱贊");
?99?
100?????????final?Label?formatsqlresult10Label?=?new?Label(shell,?SWT.NONE);
101?????????final?FormData?fd_formatsqlresult10Label?=?new?FormData();
102?????????fd_formatsqlresult10Label.top?=?new?FormAttachment(100,?-19);
103?????????fd_formatsqlresult10Label.right?=?new?FormAttachment(0,?180);
104?????????fd_formatsqlresult10Label.bottom?=?new?FormAttachment(100,?-4);
105?????????fd_formatsqlresult10Label.left?=?new?FormAttachment(0,?5);
106?????????formatsqlresult10Label.setLayoutData(fd_formatsqlresult10Label);
107?????????formatsqlresult10Label.setText("FormatSqlResult?version?2.0");
108?
109?????????final?Button?button?=?new?Button(shell,?SWT.NONE);
110?????????button.addSelectionListener(new?SelectionAdapter()?{
111?????????????public?void?widgetSelected(SelectionEvent?e)?{
112?????????????????text.selectAll();
113?????????????}
114?????????});
115?????????final?FormData?fd_button?=?new?FormData();
116?????????fd_button.top?=?new?FormAttachment(formatButton,?-22,?SWT.BOTTOM);
117?????????fd_button.bottom?=?new?FormAttachment(formatButton,?0,?SWT.BOTTOM);
118?????????fd_button.left?=?new?FormAttachment(0,?239);
119?????????fd_button.right?=?new?FormAttachment(0,?275);
120?????????button.setLayoutData(fd_button);
121?????????button.setText("全選");
122?
123?????????final?Button?button_1?=?new?Button(shell,?SWT.NONE);
124?????????button_1.addSelectionListener(new?SelectionAdapter()?{
125?????????????public?void?widgetSelected(SelectionEvent?e)?{
126?????????????????text.setText("");
127?????????????}
128?????????});
129?????????final?FormData?fd_button_1?=?new?FormData();
130?????????fd_button_1.left?=?new?FormAttachment(formatButton,?9,?SWT.DEFAULT);
131?????????fd_button_1.right?=?new?FormAttachment(100,?-242);
132?????????fd_button_1.bottom?=?new?FormAttachment(100,?-4);
133?????????fd_button_1.top?=?new?FormAttachment(100,?-26);
134?????????button_1.setLayoutData(fd_button_1);
135?????????button_1.setText("清空");
136?????}
137?
138?????public?String?getSpaceText(String?allStr)?{
139?????????String[]?strs?=?allStr.split("\n");
140?????????String?lineStr;
141?????????int?row?=?-1;
142?????????if?(strs?!=?null?&&?strs.length?>?0)?{
143?????????????lineStr?=?strs[0];
144?????????????String[]?lineStrs?=?lineStr.split("\t");
145?????????????row?=?lineStrs.length;
146?????????}
147?????????int[]?max?=?new?int[row];
148?????????for?(int?i?=?0;?i?<?max.length;?i++)?{
149?????????????max[i]?=?-1;
150?????????}
151?????????LinkedList?all?=?new?LinkedList();
152?????????for?(int?i?=?0;?i?<?row;?i++)?{
153?????????????LinkedList?list?=?new?LinkedList();
154?????????????all.add(list);
155?????????}
156?????????for?(int?i?=?0;?i?<?strs.length;?i++)?{
157?????????????lineStr?=?strs[i];
158?????????????String[]?lineStrs?=?lineStr.split("\t");
159?????????????int?length?=?-1;
160?????????????for?(int?j?=?0;?j?<?row;?j++)?{
161?????????????????if?(j?<?lineStrs.length)?{
162?????????????????????length?=?lineStrs[j].getBytes().length;
163?????????????????????if?(length?>?max[j])?{
164?????????????????????????max[j]?=?length;
165?????????????????????}
166?????????????????????((LinkedList)?all.get(j)).add(lineStrs[j]);
167?????????????????}?else?{
168?????????????????????((LinkedList)?all.get(j)).add("");
169?????????????????}
170?
171?????????????}
172?????????}
173?
174?????????StringBuffer?sb?=?new?StringBuffer();
175?????????int?line?=?((LinkedList)?all.get(0)).size();
176?????????for?(int?i?=?0;?i?<?line;?i++)?{
177?????????????for?(int?j?=?0;?j?<?all.size();?j++)?{
178?????????????????String?str?=?(String)?((LinkedList)?all.get(j)).get(i);
179?????????????????int?length?=?max[j]?-?str.getBytes().length?+?1;
180?????????????????sb.append(str);
181?????????????????for?(int?k?=?0;?k?<?length;?k++)?{
182?????????????????????sb.append("?");
183?????????????????}
184?????????????}
185?????????????sb.append("\n");
186?????????}
187?????????return?sb.toString();
188?????}
189?
190?}
191?
已制作成exe,可以雙擊執行,只支持Windows,為了兼容jdk版本,沒敢用泛型。
下載:FormatSqlResult2.rar
posted on 2007-08-10 09:55
交口稱贊 閱讀(672)
評論(1) 編輯 收藏 所屬分類:
Eclipse RCP SWT 、
java相關