#
@Min
and @Max
are used for validating numeric fields which could be String
(representing number), int
, short
, byte
etc and their respective primitive wrappers.
@Size
is used to check the length constraints on the fields.
As per documentation @Size
supports String
, Collection
, Map
and arrays
while @Min
and @Max
supports primitives and their wrappers. See the documentation.
手動觸發(fā):
https://blog.csdn.net/justyman/article/details/89857577
如果是自動觸發(fā)BUILD時,則可以以最新建立的TAG為基礎進行BUILD,而無需人手選TAG進行BUILD。
配置,注意應取消參數化配置工程:
- Add the following refspec to the Git plugin:
+refs/tags/*:refs/remotes/origin/tags/*
- Add the following branch specifier:
*/tags/*
- Enable SCM polling, so that the job detects new tags.
定義一個事件,因SPRING中可以有不同的事件,需要定義一個類以作區(qū)分:
import lombok.Getter;
import org.springframework.context.ApplicationEvent;
@Getter
public class JavaStackEvent extends ApplicationEvent {
/**
* Create a new {@code ApplicationEvent}.
*
* @param source the object on which the event initially occurred or with
* which the event is associated (never {@code null})
*/
public JavaStackEvent(Object source) {
super(source);
}
}
定義一個此事件觀察者,即感興趣者:
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import org.springframework.context.ApplicationListener;
import org.springframework.scheduling.annotation.Async;
/**
* 觀察者:讀者粉絲
*/
@RequiredArgsConstructor
public class ReaderListener implements ApplicationListener<JavaStackEvent> {
@NonNull
private String name;
private String article;
@Async
@Override
public void onApplicationEvent(JavaStackEvent event) {
// 更新文章
updateArticle(event);
}
private void updateArticle(JavaStackEvent event) {
this.article = (String) event.getSource();
System.out.printf("我是讀者:%s,文章已更新為:%s\n", this.name, this.article);
}
}
注冊感興趣者(將自身注入SPRING容器則完成注冊),并制定發(fā)布機制(通過CONTEXT發(fā)布事件):
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.CommandLineRunner;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Slf4j
@Configuration
public class ObserverConfiguration {
@Bean
public CommandLineRunner commandLineRunner(ApplicationContext context) {
return (args) -> {
log.info("發(fā)布事件:什么是觀察者模式?");
context.publishEvent(new JavaStackEvent("什么是觀察者模式?"));
};
}
@Bean
public ReaderListener readerListener1(){
return new ReaderListener("小明");
}
@Bean
public ReaderListener readerListener2(){
return new ReaderListener("小張");
}
@Bean
public ReaderListener readerListener3(){
return new ReaderListener("小愛");
}
}
Excel 在讀取 csv 的時候是通過讀取文件頭上的 bom 來識別編碼的,這導致如果我們生成 csv 文件的平臺輸出無 bom 頭編碼的 csv 文件(例如 utf-8 ,在標準中默認是可以沒有 bom 頭的),Excel 只能自動按照默認編碼讀取,不一致就會出現亂碼問題了。
掌握了這點相信亂碼已經無法阻擋我們前進的步伐了:只需將不帶 bom 頭編碼的 csv 文件,用文本編輯器(工具隨意,推薦 notepad++ )打開并轉換為帶 bom 的編碼形式(具體編碼方式隨意),問題解決。
當然,如果你是像我一樣的碼農哥哥,在生成 csv 文件的時候寫入 bom 頭更直接點,用戶會感謝你的。
附錄:對于 utf-8 編碼,unicode 標準中是沒有 bom 定義的,微軟在自己的 utf-8 格式的文本文件之前加上了EF BB BF三個字節(jié)作為識別此編碼的 bom 頭,這也解釋了為啥大部分亂碼都是 utf-8 編碼導致的原因
SPRING BATCH中生成CSV文件時的解決方案:
new FlatFileItemWriterBuilder<T>()
.name(itemWriterName)
.resource(outputResource)
.lineAggregator(lineAggregator)
.headerCallback(
h -> {
System.out.println(header);
h.write('\uFEFF');//只需加這一行
h.write(header);
}
)
.build();
https://stackoverflow.com/questions/48952319/send-csv-file-encoded-in-utf-8-with-bom-in-java
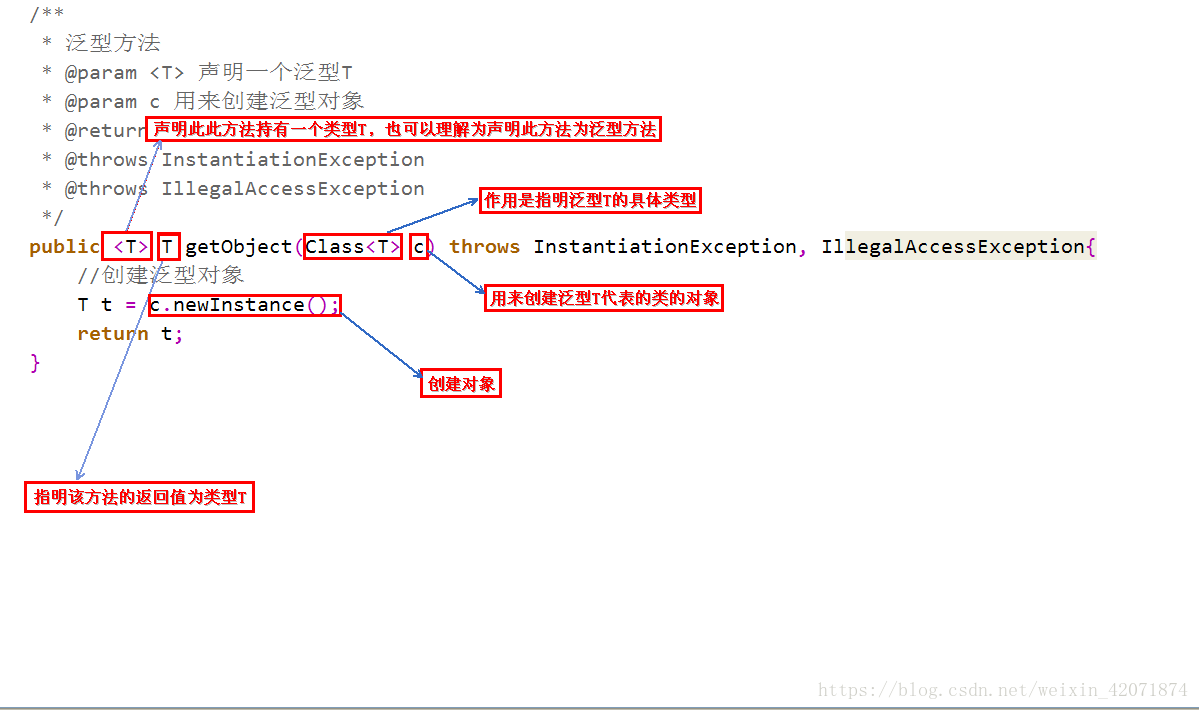
/**
*
* @param <T>聲明此方法持有一個類型T,也可以理解為聲明此方法為泛型方法
* @param clazz 作用是指明泛型T的具體類型
* @return 指明該方法的返回值為類型T
* @throws InstantiationException
* @throws IllegalAccessException
*/
public <T> T getObject(Class<T> clazz) throws InstantiationException, IllegalAccessException {
T t = clazz.newInstance();//創(chuàng)建對象
return t;
}
方法返回值前的<T>的左右是告訴編譯器,當前的方法的值傳入類型可以和類初始化的泛型類不同,也就是該方法的泛型類可以自定義,不需要跟類初始化的泛型類相同
領域驅動(DDD,Domain Driven Design)為軟件設計提供了一套完整的理論指導和落地實踐,通過戰(zhàn)略設計和戰(zhàn)術設計,將技術實現與業(yè)務邏輯分離,來應對復雜的軟件系統(tǒng)。本系列文章準備以實戰(zhàn)的角度來介紹 DDD,首先編寫領域驅動的代碼模型,然后再基于代碼模型,引入 DDD 的各項概念,先介紹戰(zhàn)術設計,再介紹戰(zhàn)略設計。
> DDD 實戰(zhàn)1 - 基礎代碼模型
> DDD 實戰(zhàn)2 - 集成限界上下文(Rest & Dubbo)
> DDD 實戰(zhàn)3 - 集成限界上下文(消息模式)
> DDD 實戰(zhàn)4 - 領域事件的設計與使用
> DDD 實戰(zhàn)5 - 實體與值對象
> DDD 實戰(zhàn)6 - 聚合的設計
> DDD 實戰(zhàn)7 - 領域工廠與領域資源庫
> DDD 實戰(zhàn)8 - 領域服務與應用服務
> DDD 實戰(zhàn)9 - 架構設計
> DDD 實戰(zhàn)10 - 戰(zhàn)略設計