Posted on 2010-11-06 17:24
セ軍魂ミ 閱讀(322)
評論(0) 編輯 收藏 所屬分類:
java技術io編程
按選項選擇操作的io小程序
1)、 在主程序中就調用一個Menu方法;
public class Main {
public static void main(String[] args){
new Menu();
}
}
2)、在vo包里包裝一個Person類,并實現Serializable接口, 且定義四個屬性:姓名,學號,年齡,成績;
3)、在op包里建立兩個類,分別為 FileOperate和InputData,前者主要實現了文件的讀、寫即保存和輸出;后者主要構建了兩個方法,一個是字符串的輸入 ,另一個是整數的輸入,并判斷輸入的是否為真。
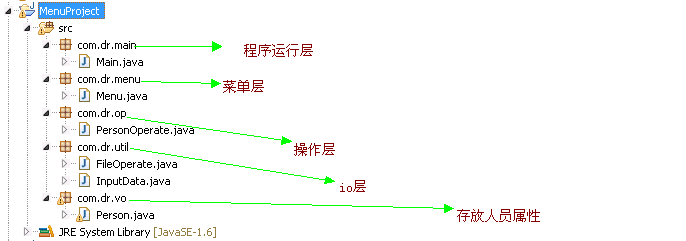
public class FileOperate {
public static final String FILENAME="d:\\person.ser";
//把對象保存在文件之中
public void save(Object obj){
ObjectOutputStream out = null;
try{
out = new ObjectOutputStream(new FileOutputStream(new File(FILENAME)));
//寫入對象
out.writeObject(obj);
}catch(Exception e){
try{
throw e;
}catch(Exception e1){}
}
finally{
try{
out.close();
}catch(Exception e){}
}
}
//把對象從文件中讀出來
public Object read() throws Exception{
Object obj = null;
ObjectInputStream input = null;
try{
input = new ObjectInputStream(new FileInputStream(new File(FILENAME)));
obj = input.readObject();
}catch(Exception e){
throw e;
}
finally{
try{
input.close();
}catch(Exception e){}
}
return obj;
}
}
public class InputData {
private BufferedReader buf = null;
//將字節的輸入流量變為字符流,之后放入緩沖之中
public InputData(){
buf = new BufferedReader(new InputStreamReader(System.in));
};
public String getString(){
String str = null;
try{
str=buf.readLine();
}catch(IOException e){}
return str;
}
public int getInt(){
int temp=0;
//如果輸入的不是數字,至少應該有一個提示,告訴用戶輸入錯了
//可以使用正則驗證
String str = null;
boolean flag = true;
while(flag){
//輸入數據
str = this.getString();
if(!(str.matches("\\d+"))){
//如果輸入的不是一個數字,則必須重新輸入
System.out.print("輸入的內容必須是整數,請你重新輸入:");
}
else{
//輸入的是一個正確的數字,則可以進行轉換
temp=Integer.parseInt(str);
//表示退出循環
flag = false;
}
}
return temp;
}
}
4)、在Menu類里建立控制臺的初始信息;
public class Menu {
InputData input = null ;
public Menu(){
this.input=new InputData();
//循環出現菜單
while(true){
this.show();
}
}
//需要定義的菜單內容
public void show(){
System.out.println("\t\t 1、增加人員信息");
System.out.println("\t\t 2、瀏覽人員信息"); ;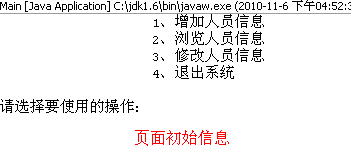
System.out.println("\t\t 3、修改人員信息");
System.out.println("\t\t 4、退出系統");
System.out.print("\n請選擇要使用的操作:");
int temp = input.getInt();
switch(temp){
case 1:{
new PersonOperate().add();
break;
}
case 2:{
new PersonOperate().show();
break;
}
case 3:{
new PersonOperate().update();
break;
}
case 4:{
System.out.println("選擇的是退出系統");
System.out.println("系統退出");
System.exit(1);
}
default: {
System.out.println("你輸入的內容不正確");
break;
}
}
}
}
5)、在PersonOperate類中進行數據的具體操作,完成最終結果的顯示即核心;
import com.dr.util.FileOperate;
import com.dr.util.InputData;
import com.dr.vo.Person;
public class PersonOperate {
private InputData input =null;
public PersonOperate(){
this.input=new InputData();
}
//完成具體的Person對象操作
public void add(){
//要使用輸入數據的類
String name = null;
String id = null;
int age = 0;
int score = 0;
System.out.print("輸入姓名為:");
name = this.input.getString();
System.out.print("輸入學號為:");
id = this.input.getString();
System.out.print("輸入年齡為:");
age = this.input.getInt();
System.out.print("輸入成績為:");
score = this.input.getInt();
//生成Person對象,把對象保存在文件中
Person p = new Person(name,id,age,score);
try{
new FileOperate().save(p); //io操作層
System.out.println("數據保存成功!");
}catch(Exception e){
System.out.println("數據保存失敗!");
}
}
public void show(){
//從文件中把內容讀進來
Person p = null;
try{
p = (Person) new FileOperate().read();
}catch(Exception e){
System.out.println("內容顯示失敗,請確定數據是否存在!");
}
if(p!=null){
System.out.println(p);
}
}
public void update(){
//先將之前的信息查出來
Person p = null;
try{
p = (Person) new FileOperate().read();
}catch(Exception e){
System.out.println("內容顯示失敗,請確定數據是否存在!");
}
if(p!=null){
String name = null;
String id= null;
int age = 0;
int score=0;
System.out.print("請輸入新的姓名(原姓名為:"+p.getName()+")");
name = this.input.getString();
System.out.print("請輸入新的學號(原學號為:"+p.getId()+")");
id = this.input.getString();
System.out.print("請輸入新的年齡(原年齡為:"+p.getAge()+")");
age = this.input.getInt();
System.out.print("請輸入新的成績(原成績為:"+p.getScore()+")");
score = this.input.getInt();
//信息重新設置
p.setName(name);
p.setId(id);
p.setAge(age);
p.setScore(score);
try{
new FileOperate().save(p);
System.out.println("數據更新成功!");
}catch(Exception e){
System.out.println("數據更新失敗!");
}
}
}
}
6)、程序最后的運行結果及方式:
最后希望大家給予點評,我好做修改!謝謝!