摘要: 制作一個簡單的Grid
1var cm = new Ext.grid.ColumnModel([
2 {header:'編號',dataIndex:'id'},
3 {header:'名稱',dataIndex:'name...
閱讀全文
摘要: 本文轉載于javaeye(http://www.javaeye.com/wiki/Object_Oriented_JavaScript/1279-javascript-object-oriented-technology-one),只進行了重新排版以便收藏。
&nb...
閱讀全文
本文轉載于javaeye(
http://www.javaeye.com/wiki/Object_Oriented_JavaScript/1279-javascript-object-oriented-technology-one),只進行了重新排版以便收藏。
文中所有英文語句(程序語句除外),都引自<<javascript-the definitive guide,5th edition>>。
------------------------------------------------------------------------------------
類變量/類方法/實例變量/實例方法
先補充一下以前寫過的方法:
在javascript中,所有的方法都有一個call方法和apply方法。這兩個方法可以模擬對象調用方法。它的第一個參數是對象,后面的參數表示對象調用這個方法時的參數(ECMAScript specifies two methods that are defined for all functions, call() and apply(). These methods allow you to invoke a function as if it were a method of some other object. The first argument to both call() and apply() is the object on which the function is to be invoked; this argument becomes the value of the this keyword within the body of the function. Any remaining arguments to call() are the values that are passed to the function that is invoked)。比如我們定義了一個方法f(),然后調用下面的語句:
f.call(o, 1, 2);
作用就相當于
o.m = f;
o.m(1,2);
delete o.m;
舉個例子:
1
function Person(name,age)
{ //定義方法
2
this.name = name;
3
this.age = age;
4
}
5
var o = new Object(); //空對象
6
alert(o.name + "_" + o.age); //undefined_undefined
7
8
Person.call(o,"sdcyst",18); //相當于調用:o.Person("sdcyst",18)
9
alert(o.name + "_" + o.age); //sdcyst_18
10
11
Person.apply(o,["name",89]);//apply方法作用同call,不同之處在于傳遞參數的形式是用數組來傳遞
12
alert(o.name + "_" + o.age); //name_89
---------------------------------
實例變量和實例方法都是通過實例對象加"."操作符然后跟上屬性名或方法名來訪問的,但是我們也可以為類來設置方法或變量,
這樣就可以直接用類名加"."操作符然后跟上屬性名或方法名來訪問。定義類屬性和類方法很簡單:
1
Person.counter = 0; //定義類變量,創建的Person實例的個數
2
function Person(name,age)
{
3
this.name = name;
4
this.age = age;
5
Person.counter++; //沒創建一個實例,類變量counter加1
6
};
7
8
Person.whoIsOlder = function(p1,p2)
{ //類方法,判斷誰的年齡較大
9
if(p1.age > p2.age)
{
10
return p1;
11
} else
{
12
return p2;
13
}
14
}
15
16
var p1 = new Person("p1",18);
17
var p2 = new Person("p2",22);
18
19
alert("現在有 " + Person.counter + "個人"); //現在有2個人
20
var p = Person.whoIsOlder(p1,p2);
21
alert(p.name + "的年齡較大"); //p2的年齡較大
prototype屬性的應用:
下面這個例子是根據原書改過來的.
假設我們定義了一個Circle類,有一個radius屬性和area方法,實現如下:
1
function Circle(radius)
{
2
this.radius = radius;
3
this.area = function()
{
4
return 3.14 * this.radius * this.radius;
5
}
6
}
7
var c = new Circle(1);
8
alert(c.area()); //3.14
假設我們定義了100個Circle類的實例對象,那么每個實例對象都有一個radius屬性和area方法。實際上,除了radius屬性,每個Circle類的實例對象的area方法都是一樣,這樣的話,我們就可以把area方法抽出來定義在Circle類的prototype屬性中,這樣所有的實例對象就可以調用這個方法,從而節省空間。
1
function Circle(radius)
{
2
this.radius = radius;
3
}
4
Circle.prototype.area = function()
{
5
return 3.14 * this.radius * this.radius;
6
}
7
var c = new Circle(1);
8
alert(c.area()); //3.14
現在,讓我們用prototype屬性來模擬一下類的繼承:首先定義一個Circle類作為父類,然后定義子類PositionCircle。
1
function Circle(radius)
{ //定義父類Circle
2
this.radius = radius;
3
}
4
Circle.prototype.area = function()
{ //定義父類的方法area計算面積
5
return this.radius * this.radius * 3.14;
6
}
7
8
function PositionCircle(x,y,radius)
{ //定義類PositionCircle
9
this.x = x; //屬性橫坐標
10
this.y = y; //屬性縱坐標
11
Circle.call(this,radius); //調用父類的方法,相當于調用this.Circle(radius),設置PositionCircle類的
12
//radius屬性
13
}
14
PositionCircle.prototype = new Circle(); //設置PositionCircle的父類為Circle類
15
16
var pc = new PositionCircle(1,2,1);
17
alert(pc.area()); //3.14
18
//PositionCircle類的area方法繼承自Circle類,而Circle類的
19
//area方法又繼承自它的prototype屬性對應的prototype對象
20
alert(pc.radius); //1 PositionCircle類的radius屬性繼承自Circle類
21
22
/**//*
23
注意:在前面我們設置PositionCircle類的prototype屬性指向了一個Circle對象,
24
因此pc的prototype屬性繼承了Circle對象的prototype屬性,而Circle對象的constructor屬
25
性(即Circle對象對應的prototype對象的constructor屬性)是指向Circle的,所以此處彈出
26
的是Circ.
27
*/
28
alert(pc.constructor); //Circle
29
30
/**//*為此,我們在設計好了類的繼承關系后,還要設置子類的constructor屬性,否則它會指向父類
31
的constructor屬性
32
*/
33
PositionCircle.prototype.constructor = PositionCircle
34
alert(pc.constructor); //PositionCircle
本文轉載于javaeye(
http://www.javaeye.com/wiki/Object_Oriented_JavaScript/1279-javascript-object-oriented-technology-one),只進行了重新排版以便收藏。
文中所有英文語句(程序語句除外),都引自<<javascript-the definitive guide,5th edition>>。
------------------------------------------------------------------------------------
類、構造函數、原型
先來說明一點:在上面的內容中提到,每一個函數都包含了一個prototype屬性,這個屬性指向了一個prototype對象(Every function has a prototype property that refers to a predefined prototype object --section8.6.2)。注意不要搞混了。
構造函數:
new操作符用來生成一個新的對象。new后面必須要跟上一個函數,也就是我們常說的構造函數。構造函數的工作原理又是怎樣的呢?先看一個例子:
1
function Person(name,sex)
{
2
this.name = name;
3
this.sex = sex;
4
}
5
var per = new Person("sdcyst","male");
6
alert("name:"+per.name+"_sex:"+per.sex); //name:sdcyst_sex:male
下面說明一下這個工作的步驟:
開始創建了一個函數(不是方法,只是一個普通的函數),注意用到了this關鍵字。以前我們提到過this關鍵字表示調用該方法的對象,也就是說通過對象調用"方法"的時候,this關鍵字會指向該對象(不使用對象直接調用該函數則this指向整個的script域,或者函數所在的域,在此我們不做詳細的討論)。當我們使用new操作符時,javascript會先創建一個空的對象,然后這個對象被new后面的方法(函數)的this關鍵字引用!然后在方法中通過操作this,就給這個新創建的對象相應的賦予了屬性。最后返回這個經過處理的對象。這樣上面的例子就很清楚:先創建一個空對象,然后調用Person方法對其進行賦值,最后返回該對象,我們就得到了一個per對象。
prototype(原型)--在這里會反復提到"原型對象"和"原型屬性",注意區分這兩個概念。
在javascript中,每個對象都有一個prototype屬性,這個屬性指向了一個prototype對象。上面我們提到了用new來創建一個對象的過程,事實上在這個過程中,當創建了空對象后,new會接著操作剛生成的這個對象的prototype屬性。每個方法都有一個prototype屬性(因為方法本身也是對象),new操作符生成的新對象的prototype屬性值和構造方法的prototype屬性值是一致的。構造方法的prototype屬性指向了一個prototype對象,這個prototype對象初始只有一個屬性constructor,而這個constructor屬性又指向了prototype屬性所在的方法(In the previous section, I showed that the new operator creates a new, empty object and then invokes a constructor function as a method of that object. This is not the complete story, however. After creating the empty object, new sets the prototype of that object. The prototype of an object is the value of the prototype property of its constructor function. All functions have a prototype property that is automatically created and initialized when the function is defined. The initial value of the prototype property is an object with a single property. This property is named constructor and refers back to the constructor function with which the prototype is associated. this is why every object has a constructor property. Any properties you add to this prototype object will appear to be properties of objects initialized by the constructor. -----section9.2)
有點暈,看下面的圖:
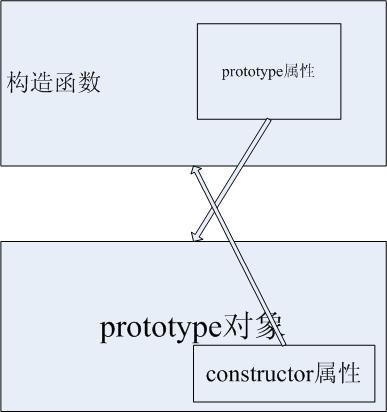
這樣,當用構造函數創建一個新的對象時,它會獲取構造函數的prototype屬性所指向的prototype對象的所有屬性。對構造函數對應的prototype對象所做的任何操作都會反應到它所生成的對象身上,所有的這些對象共享構造函數對應的prototype對象的屬性(包括方法)。看個具體的例子吧:
1
function Person(name,sex)
{ //構造函數
2
this.name = name;
3
this.sex = sex;
4
}
5
Person.prototype.age = 12; //為prototype屬性對應的prototype對象的屬性賦值
6
Person.prototype.print = function()
{ //添加方法
7
alert(this.name+"_"+this.sex+"_"+this.age);
8
};
9
10
var p1 = new Person("name1","male");
11
var p2 = new Person("name2","male");
12
p1.print(); //name1_male_12
13
p2.print(); //name2_male_12
14
15
Person.prototype.age = 18; //改變prototype對象的屬性值,注意是操作構造函數的prototype屬性
16
p1.print(); //name1_male_18
17
p2.print(); //name2_male_18
到目前為止,我們已經模擬出了簡單的類的實現,我們有了構造函數,有了類屬性,有了類方法,可以創建"實例"。在下面的文章中,我們就用"類"這個名字來代替構造方法,但是,這僅僅是模擬,并不是真正的面向對象的"類"。在下一步的介紹之前,我們先來看看改變對象的prototype屬性和設置prototype屬性的注意事項:給出一種不是很恰當的解釋,或許有助于我們理解:當我們new了一個對象之后,這個對象就會獲得構造函數的prototype屬性(包括函數和變量),可以認為是構造函數(類)繼承了它的prototype屬性對應的prototype對象的函數和變量,也就是說,
prototype對象模擬了一個超類的效果。聽著比較拗口,我們直接看個實例吧:
1
function Person(name,sex)
{ //Person類的構造函數
2
this.name = name;
3
this.sex = sex;
4
}
5
Person.prototype.age = 12; //為Person類的prototype屬性對應的prototype對象的屬性賦值,
6
//相當于為Person類的父類添加屬性
7
Person.prototype.print = function()
{ //為Person類的父類添加方法
8
alert(this.name+"_"+this.sex+"_"+this.age);
9
};
10
11
var p1 = new Person("name1","male"); //p1的age屬性繼承子Person類的父類(即prototype對象)
12
var p2 = new Person("name2","male");
13
14
p1.print(); //name1_male_12
15
p2.print(); //name2_male_12
16
17
p1.age = 34; //改變p1實例的age屬性
18
p1.print(); //name1_male_34
19
p2.print(); //name2_male_12
20
21
Person.prototype.age = 22; //改變Person類的超類的age屬性
22
p1.print(); //name1_male_34(p1的age屬性并沒有隨著prototype屬性的改變而改變)
23
p2.print(); //name2_male_22(p2的age屬性發生了改變)
24
25
p1.print = function()
{ //改變p1對象的print方法
26
alert("i am p1");
27
}
28
29
p1.print(); //i am p1(p1的方法發生了改變)
30
p2.print(); //name2_male_22(p2的方法并沒有改變)
31
32
Person.prototype.print = function()
{ //改變Person超類的print方法
33
alert("new print method!");
34
}
35
36
p1.print(); //i am p1(p1的print方法仍舊是自己的方法)
37
p2.print(); //new print method!(p2的print方法隨著超類方法的改變而改變)
看過一篇文章介紹說javascript中對象的prototype屬性相當于java中的static變量,可以被這個類下的所有對象共用。而上面的例子似乎表明實際情況并不是這樣:在JS中,當我們用new操作符創建了一個類的實例對象后,它的方法和屬性確實繼承了類的prototype屬性,類的prototype屬性
中定義的方法和屬性,確實可以被這些實例對象直接引用。但是,當我們對這些實例對象的屬性和方法重新賦值或定義后,那么實例對象的屬性或方法就不再指向類的prototype屬性中定義的屬性和方法。此時,即使再對類的prototype屬性中相應的方法或屬性做修改,也不會反應在實例對象身上。這就解釋了上面的例子:一開始,用new操作符生成了兩個對象p1,p2,他們的age屬性和print方法都來自(繼承于)Person類的prototype屬性.然后,我們修改了p1的age屬性,后面對Person類的prototype屬性中的age重新賦值(Person.prototype.age = 22),p1的age屬性并不會隨之改變,但是p2的age屬性卻隨之發生了變化,因為p2的age屬性還是引自Person類的prototype屬性。同樣的情況在后面的print方法中也體現了出來。
通過上面的介紹,我們知道prototype屬性在javascript中模擬了父類(超類)的角色,在js中體現面向對象的思想,prototype屬性是非常關鍵的。
本文轉載于javaeye(
http://www.javaeye.com/wiki/Object_Oriented_JavaScript/1279-javascript-object-oriented-technology-one),只進行了重新排版以便收藏。
文中所有英文語句(程序語句除外),都引自<<javascript-the definitive guide,5th edition>>。
------------------------------------------------------------------------------------
函數
javascript函數相信大家都寫過不少了,所以我們這里只是簡單介紹一下。
創建函數:
function f(x) {........}
var f = function(x) {......}
上面這兩種形式都可以創建名為f()的函數,不過后一種形式可以創建匿名函數。函數定義時可以設置參數,如果傳給函數的參數個數不夠,則從最左邊起依次對應,其余的用undefined賦值,如果傳給函數的參數多于函數定義參數的個數,則多出的參數被忽略。
1
function myprint(s1,s2,s3)
{
2
alert(s1+"_"+s2+"_"+s3);
3
}
4
myprin(); //undefined_undefined_undefined
5
myprint("string1","string2"); //string1_string2_undefined
6
myprint("string1","string2","string3","string4"); //string1_string2_string3
因此,對于定義好的函數,我們不能指望調用者將所有的參數全部傳進來。對于那些必須用到的參數應該在函數體中加以檢測(用!操作符),或者設置默認值然后同參數進行或(||)操作來取得參數。
1
function myprint(s1,person)
{
2
var defaultperson =
{ //默認person對象
3
"name":"name1",
4
"age":18,
5
"sex":"female"
6
};
7
if(!s1)
{ //s1不允許為空
8
alert("s1 must be input!");
9
return false;
10
}
11
person = person || defaultperson; //接受person對象參數
12
alert(s1+"_"+person.name+":"+person.age+":"+person.sex);
13
};
14
15
myprint(); //s1 must be input!
16
myprint("s1"); //s1_name1:18:female
17
myprint("s1",
{"name":"sdcyst","age":23,"sex":"male"}); //s1_sdcyst:23:male
函數的arguments屬性
在每一個函數體的內部,都有一個arguments標識符,這個標識符代表了一個Arguments對象。Arguments對象非常類似于Array(數組)對象,比如都有length屬性,訪問它的值用"[]"操作符利用索引來訪問參數值。但是,二者是完全不同的東西,僅僅是表面上有共同點而已(比如說修改Arguments對象的length屬性并不會改變它的長度)。
1
function myargs()
{
2
alert(arguments.length);
3
alert(arguments[0]);
4
}
5
myargs(); //0 --- undefined
6
myargs("1",[1,2]); //2 --- 1
Arguments對象有一個callee屬性,標示了當前Arguments對象所在的方法。可以使用它來實現匿名函數的內部遞歸調用。
1
function(x)
{
2
if (x <= 1) return 1;
3
return x * arguments.callee(x-1);
4
} (section8.2)
------------------------------------------------------------------
Method--方法
方法就是函數。我們知道,每一個對象都包含0個或多個屬性,屬性可以是任意類型,當然也包括對象。函數本身就是一種對象,因此我們完全可以把一個函數放到一個對象里面,此時,這個函數就成了對象的一個方法。此后如果要使用該方法,則可以通過對象名利用"."操作符來實現。
1
var obj =
{f0:function()
{alert("f0");}}; //對象包含一個方法
2
function f1()
{alert("f1");}
3
obj.f1 = f1; //為對象添加方法
4
5
obj.f0(); //f0 f0是obj的方法
6
obj.f1(); //f1 f1是obj的方法
7
f1(); //f1 f1同時又是一個函數,可以直接調用
8
f0(); //f0僅僅是obj的方法,只能通過對象來調用
方法的調用需要對象的支持,那么在方法中如何獲取對象的屬性呢?this!this關鍵字我們已經很熟悉了,在javascript的方法中,我們可以用this來取得對方法調用者(對象)的引用,從而獲取方法調用者的各種屬性。
1
var obj =
{"name":"NAME","sex":"female"};
2
obj.print = function()
{ //為對象添加方法
3
alert(this.name + "_" + this["sex"]);
4
};
5
obj.print(); //NAME_female
6
obj.sex = "male";
7
obj.print(); //NAME_male
下面我們來一個更加面向對象的例子:
1
var person =
{name:"defaultname",
2
setName:function(s)
{
3
this.name = s;
4
},
5
"printName":function()
{
6
alert(this.name);
7
}}
8
person.printName(); //defaultname
9
person.setName("newName");
10
person.printName(); //newName
在上面的例子中,完全可以用person.name=..來直接改變person的name屬性,在此我們只是為了展示一下剛才提到的內容。
另一種改變person屬性的方法就是:定義一個function,接收兩個參數,一個是person,一個是name的值,看起來像是這樣:changeName(person,"newName")。哪種方法好呢?很明顯,例子中的方法更形象,更直觀一些,而且好像有了那么一點面向對象的影子。
再次強調一下,方法(Method)本身就是是函數(function),只不過方法的使用更受限制。在后面的篇幅中,如果提到函數,那么提到的內容同樣適用于方法,反之則不盡然。
函數的prototype屬性
每一個函數都包含了一個prototype(原型)屬性,這個屬性構成了javascript面向對象的核心基礎。在后面我們會詳細討論。
本文轉載于javaeye(
http://www.javaeye.com/wiki/Object_Oriented_JavaScript/1279-javascript-object-oriented-technology-one),只進行了重新排版以便收藏。
文中所有英文語句(程序語句除外),都引自<<javascript-the definitive guide,5th edition>>。
------------------------------------------------------------------------------------
數組
我們已經提到過,對象是無序數據的集合,而數組則是有序數據的集合,數組中的數據(元素)通過索引(從0開始)來訪問,數組中的數據可以是任何的數據類型。數組本身仍舊是對象,但是由于數組的很多特性,通常情況下把數組和對象區別開來分別對待(Throughout this book, objects and arrays are often treated as distinct datatypes. This is a useful and reasonable simplification; you can treat objects and arrays as separate types for most of your JavaScript programming.To fully understand the behavior of objects and arrays, however, you have to know the truth: an array is nothing more than an object with a thin layer of extra functionality. You can see this with the typeof operator: applied to an array value, it returns the string "object". --section7.5).
創建數組可以用"[]"操作符,或者是用Array()構造函數來new一個。
1
var array1 = []; //創建空數組
2
var array2 = new Array(); //創建空數組
3
array1 = [1,"s",[3,4],
{"name1":"NAME1"}]; //
4
alert(array1[2][1]); //4 訪問數組中的數組元素
5
alert(array1[3].name1); //NAME1 訪問數組中的對象
6
alert(array1[8]); //undefined
7
array2 = [,,]; //沒有數值填入只有逗號,則對應索引處的元素為undefined
8
alert(array2.length); //3
9
alert(array2[1]); //undefined
用new Array()來創建數組時,可以指定一個默認的大小,其中的值此時為undefined,以后可以再給他們賦值.但是由于javascript中的數組的長度是可以任意改變的,同時數組中的內容也是可以任意改變的,因此這個初始化的長度實際上對數組沒有任何的約束力。對于一個數組,如果對超過它最大長度的索引賦值,則會改變數組的長度,同時會對沒有賦值
的索引處賦值undefined,看下面的例子:
1
var array = new Array(10);
2
alert(array.length); //10
3
alert(array[4]); //undefined
4
array[100] = "100th"; //這個操作會改變數組的長度,同時將10-99索引對應的值設為undefined
5
alert(array.length); //101
6
alert(array[87]); //undefined
可以用delete操作符刪除數組的元素,注意這個刪除僅僅是將數組在該位置的元素設為undefined,數組的長度并沒有改變。我們已經使用過了數組的length屬性,length屬性是一個可以讀/寫的屬性,也就是說我們可以通過改變數組的length屬性來任意的改變數組的長度。如果將length設為小于數組長度的值,則原數組中索引大于length-1的值都會被刪除。如果length的值大于原始數組的長度,則在它們之間的值設為undefined。
1
var array = new Array("n1","n2","n3","n4","n5"); //五個元素的數組
2
var astring = "";
3
for(var i=0; i<array.length; i++)
{ //循環數組元素
4
astring += array[i];
5
}
6
alert(astring); //n1n2n3n4n5
7
delete array[3]; //刪除數組元素的值
8
alert(array.length + "_" + array[3]) //5_undefined
9
10
array.length = 3; //縮減數組的長度
11
alert(array[3]); //undefined
12
array.length = 8; //擴充數組的長度
13
alert(array[4]); //undefined
對于數組的其他方法諸如join/reverse等等,在這就不再一一舉例。
通過上面的解釋,我們已經知道,對象的屬性值是通過屬性的名字(字符串類型)來獲取,而數組的元素是通過索引(整數型 0~~2**32-1)來得到值。數組本身也是一個對象,所以對象屬性的操作也完全適合于數組。
1
var array = new Array("no1","no2");
2
array["po"] = "props1";
3
alert(array.length); //2
4
//對于數組來說,array[0]同array["0"]效果是一樣的(?不確定,測試時如此)
5
alert(array[0] + "_" + array["1"] + "_" + array.po);//no1_no2_props1
本文轉載于javaeye(
http://www.javaeye.com/wiki/Object_Oriented_JavaScript/1279-javascript-object-oriented-technology-one),只進行了重新排版以便收藏。
文中所有英文語句(程序語句除外),都引自<<javascript-the definitive guide,5th edition>>。
------------------------------------------------------------------------------------
對象和數組(Objects and Arrays)
什么是對象?把一些"名字-屬性"的組合放在一個單元里面,就組成了一個對象。我們可以理解為javascript中的對象就是一些"鍵-值"對的集合(An object is a collection of named values. These named values are usually referred to as properties of the object.--Section3.5)。
"名字"只能是string類型,不能是其他類型,而屬性的類型則是任意的(數字/字符串/其他對象..)。可以用new Object()來創建一個空對象,也可以簡單的用"{}"來創建一個空對象,這兩者的作用是等同的。
1
var emptyObject1 =
{}; //創建空對象
2
var emptyObject2 = new Object(); //創建空對象
3
var person =
{"name":"sdcyst",
4
"age":18,
5
"sex":"male"}; //創建一個包含初始值的對象person
6
alert(person.name); //sdcyst
7
alert(person["age"]); //18
從上面的例子我們也可以看到,訪問一個對象的屬性,可以簡單的用對象名加"."后加屬性的名字,也可以用"[]"操作符來獲取,此時在[]里面的屬性名字要加引號,這是因為對象中的索引都是字符串類型的。javasript對象中屬性的個數是可變的,在創建了一個對象之后可以隨時對它賦予任何的屬性。
1
var person =
{};
2
person.name = "sdcyst";
3
person["age"] = 18;
4
alert(person.name + "__" + person.age); //sdcyst__18
5
6
var _person =
{name:"balala","age":23}; //在構建一個對象時,屬性的名字可以不用引號來標注(name),
7
//但是仍舊是一個字符串類型.在訪問的時候[]內仍舊需要引號
8
alert(_person["name"] + "__" + person.age); //balala__23
9
alert(_person[name]); //undefinied
通過"."操作符獲取對象的屬性,必須得知道屬性的名字。一般來說"[]"操作符獲取對象屬性的功能更強大一些,可以在[]中放入一些表達式來取屬性的值,比如可以用在循環控制語句中,而"."操作符則沒有這種靈活性。
1
var name =
{"name1":"NAME1","name2":"NAME2","name3":"NAME3","name4":"NAME4"};
2
var namestring = "";
3
for(var props in name)
{ //循環name對象中的屬性名字
4
namestring += name[props];
5
}
6
alert(namestring); //NAME1NAME2NAME3NAME4
7
8
namestring = "";
9
for(var i=0; i<4; i++)
{
10
namestring += name["name"+(i+1)];
11
}
12
alert(namestring); //NAME1NAME2NAME3NAME4
delete操作符可以刪除對象中的某個屬性,判斷某個屬性是否存在可以使用"in"操作符。
1
var name =
{"name1":"NAME1","name2":"NAME2","name3":"NAME3","name4":"NAME4"};
2
var namestring = "";
3
for(var props in name)
{ //循環name對象中的屬性名字
4
namestring += name[props];
5
}
6
alert(namestring); //NAME1NAME2NAME3NAME4
7
8
delete name.name1; //刪除name1屬性
9
delete name["name3"]; //刪除name3屬性
10
namestring = "";
11
for(var props in name)
{ //循環name對象中的屬性名字
12
namestring += name[props];
13
}
14
alert(namestring); //NAME2NAME4
15
16
alert("name1" in name); //false
17
alert("name4" in name); //true
需要注意,對象中的屬性是沒有順序的。
對象的constructor屬性
每一個javascript對象都有一個constructor屬性。這個屬性對應了對象初始化時的構造函數(函數也是對象)。
1
var date = new Date();
2
alert(date.constructor); //Date
3
alert(date.constructor == "Date"); //false
4
alert(date.constructor == Date); //true
在org.apache.commons.lang.math包中,一共有11個類。可以分成四組:
1. 處理分數的Fraction類;
2.處理數值的NumberUtils和IEEE754rUtils類,這里IEEE745r代表的是IEEE 754的標準,是一種浮點數的處理標準。
3.處理隨機數的JVMRandom和RandomUtils類。
4.處理數值范圍的Range, DoubleRange, FloatRange, IntRange, LangRange, NumberRange類。
Fraction類能很方便地處理分數,并能進行分數的約分,加減乘除和指數運算以及求相對值。如:

private void FractionDemo()
{
Fraction fraction = Fraction.getFraction(0.5);
System.out.println(fraction.getNumerator());
System.out.println(fraction.getDenominator());

fraction = Fraction.getFraction("1/2");
System.out.println(fraction.doubleValue());

fraction = Fraction.getFraction(1, 2);
System.out.println(fraction.doubleValue());

fraction = Fraction.getFraction(1, 1, 2);
System.out.println(fraction.doubleValue());

fraction = Fraction.getFraction(2, 4);
System.out.println(fraction.doubleValue());
fraction = Fraction.getReducedFraction(2, 4);
System.out.println(fraction.doubleValue());

System.out.println(Fraction.getFraction(-1, 2).abs());
System.out.println(Fraction.getFraction(1, -2).abs());
System.out.println(Fraction.getFraction(1, 2).add(
Fraction.getFraction(1, 2)));
System.out.println(Fraction.getFraction(1, 2).subtract(
Fraction.getFraction(1, 2)));
System.out.println(Fraction.getFraction(1, 2).multiplyBy(
Fraction.getFraction(1, 2)));
System.out.println(Fraction.getFraction(1, 2).divideBy(
Fraction.getFraction(1, 2)));
System.out.println(Fraction.getFraction(1, 2).pow(2));
}
NumberUtils的功能相對來說就有點雞肋了,他能通過createXXX(String str)創建各種類型的數值,即使你傳入的參數是0X123這樣代表16進制的數,它也能正確解析出來。同時它還具有獲取數組最大最小數的功能。

private void NumberUtilsDemo()
{
System.out.println("Is 0x3F a number? " + NumberUtils.isNumber("0x3F"));

double[] array =
{ 1.0, 3.4, 0.8, 7.1, 4.6 };
double max = NumberUtils.max(array);
double min = NumberUtils.min(array);
System.out.println("Max of array is: " + max);
System.out.println("Min of array is: " + min);
System.out.println();
}
JVMRandom繼承了java.util.Random類,其功能和Random差不多,只不過封裝了返回不同數據類型的方法而已。而RandomUtils則把JVMRandom的方法靜態化了。

private void RandomUtilsDemo()
{

for (int i = 0; i < 5; i++)
{
System.out.println(RandomUtils.nextInt(100));
}
System.out.println();
}
Range是一個abstract類,主要處理數值范圍的。DoubleRange, FloatRange, IntRange, LangRange和NumberRange都繼承了Range類,它們差不多,只是處理的數據類型不同而已。所以看一個類的使用方法就等于看了所有類的使用方法。

private void NumberRangeDemo()
{
Range normalScoreRange = new DoubleRange(90, 120);
double score1 = 102.5;
double score2 = 79.9;
System.out.println("Normal score range is: " + normalScoreRange);
System.out.println("Is " + score1 + " a normal score? " + normalScoreRange.containsDouble(score1));
System.out.println("Is " + score2 + " a normal score? " + normalScoreRange.containsDouble(score2));
System.out.println(normalScoreRange.overlapsRange(new IntRange(92,119)));
}
摘要: 在org.apache.commons.lang.builder包中一共有7個類,用于幫助實現Java對象的一些基礎的方法,如compareTo(), equals(), hashCode(), toString()等。他們分別是:
CompareToBuil...
閱讀全文