項目需求:1.病人來掛號時根據(jù)自己想找的醫(yī)生掛相應的醫(yī)生的號,即加到相應醫(yī)生的病人隊列中。
2.醫(yī)生看見自己的屏幕,點擊自己的名字,得到自己相應列表的下一位病人
具體代碼如下:
醫(yī)生:
package com.dr.queue;

import java.util.LinkedList;
import java.util.List;
import java.util.Queue;


public class Doctor
{
private String dName;
private int dNum;
private Queue<Patient> patientList;
private Patient p;


public Patient getP()
{
return p;
}


public void setP(Patient p)
{
this.p = p;
}


public Doctor(String name, int dNum)
{
this.setdName(dName);
this.setdNum(dNum);
patientList = new LinkedList<Patient>();
// this.setPatientList(new LinkedList<Patient>());
}


public Queue<Patient> getPatientList()
{
return patientList;
}

// public void setPatientList(Queue<Patient> patientList) {
// this.patientList = patientList;
// }

public String getdName()
{
return dName;
}


public void setdName(String dName)
{
this.dName = dName;
}


public int getdNum()
{
return dNum;
}


public void setdNum(int dNum)
{
this.dNum = dNum;
}


public void offerPatient(Patient patient)
{
this.patientList.offer(patient);
// return patientList;
}


public void looking()
{
System.out.println("I handling the " + this.p.getpNum() + " waiter");

try
{
Thread.sleep(100);

} catch (InterruptedException e)
{
e.printStackTrace();
}
System.out.println("I have handled the patient,next ,next ,next");

}

}

病人:
package com.dr.queue;


public class Patient
{
private String pName;
package com.dr.ui;

import java.util.Queue;

import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;

import com.dr.queue.Patient;
import com.dr.queue.QueueServer;


public class DoctorQueueUI
{

public static void main(String[] args)
{
final Display display = Display.getDefault();
final Shell shell = new Shell();
shell.setMaximized(true);
shell.setText("醫(yī)生使用客戶端");

// final Map<Doctor,List<Patient>> m = new
// HashMap<Doctor,List<Patient>>();

final Text txt = new Text(shell, SWT.MULTI);
txt.setBounds(350, 50, 500, 450);

QueueServer qs = new QueueServer();
final Queue<Patient> rosePL = qs.init("rose");
final Queue<Patient> xiaoPL = qs.init("xiaoxiao");
final Queue<Patient> yangPL = qs.init("yangguang");

final Button button1 = new Button(shell, SWT.Activate);
button1.setBounds(250, 530, 200, 75); // 設置按鈕位置
button1.setFont(new Font(display, "宋體", 12, SWT.BOLD));
button1.setText("一號專家rose");// 設置按鈕上的文字


button1.addSelectionListener(new SelectionAdapter()
{

public void widgetSelected(SelectionEvent e)
{

Patient patient = rosePL.poll();


if (patient != null)
{
txt.setText("請" + patient.getpNum() + "號病人到一號專家rose這就診!");

} else
{
txt.setText("現(xiàn)在沒有病人就診,您可以休息一下!");
}
//
}
});
final Button button2 = new Button(shell, SWT.Activate);
button2.setBounds(500, 530, 200, 75); // 設置按鈕位置
button2.setFont(new Font(display, "宋體", 12, SWT.BOLD));
button2.setText("二號專家xiaoxiao");// 設置按鈕上的文字


button2.addSelectionListener(new SelectionAdapter()
{

public void widgetSelected(SelectionEvent e)
{
Patient patient = xiaoPL.poll();

if (patient != null)
{
txt
.setText("請" + patient.getpNum()
+ "號病人到二號專家xiaoxiao這就診");

} else
{
txt.setText("現(xiàn)在沒有病人就診,您可以休息一下");
}
//
}
});
final Button button3 = new Button(shell, SWT.Activate);
button3.setBounds(750, 530, 200, 75); // 設置按鈕位置
button3.setFont(new Font(display, "宋體", 12, SWT.BOLD));
button3.setText("三好專家yangguang");// 設置按鈕上的文字


button3.addSelectionListener(new SelectionAdapter()
{

public void widgetSelected(SelectionEvent e)
{

Patient patient = yangPL.poll();


if (patient != null)
{
txt.setText("請" + patient.getpNum()
+ "號病人到三好專家yangguang這就診");

} else
{
txt.setText("現(xiàn)在沒有病人就診,您可以休息一下");
}
//
}
});

shell.layout();
shell.open();

while (!shell.isDisposed())
{
if (!display.readAndDispatch())
display.sleep();
}

}
}

private int pNum;
private int frontNum;

public int getFrontNum()
{
return frontNum;
}

public void setFrontNum(int frontNum)
{
this.frontNum = frontNum;
}

public String getpName()
{
return pName;
}

public void setpName(String pName)
{
this.pName = pName;
}

public int getpNum()
{
return pNum;
}

public void setpNum(int pNum)
{
this.pNum = pNum;
}
}

病人掛號界面UI:
package com.dr.ui;

import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Queue;

import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;

import com.dr.queue.Doctor;
import com.dr.queue.Patient;
import com.dr.queue.QueueServer;


public class PatientQueueUI
{

public static void main(String[] args)
{
final Display display = Display.getDefault();
final Shell shell = new Shell();
shell.setMaximized(true);
shell.setText("醫(yī)院排隊病人使用客戶端");

// final Map<Doctor,Queue<Patient>> m = new
// HashMap<Doctor,Queue<Patient>>();
QueueServer qs = new QueueServer();
final Queue<Patient> rosePL = qs.init("rose");
final Queue<Patient> xiaoPL = qs.init("xiaoxiao");
final Queue<Patient> yangPL = qs.init("yangguang");

final Text txt = new Text(shell, SWT.MULTI);
txt.setBounds(350, 50, 500, 450);

final Button button1 = new Button(shell, SWT.Activate);
button1.setBounds(250, 530, 200, 75); // 設置按鈕位置
button1.setFont(new Font(display, "宋體", 12, SWT.BOLD));
button1.setText("一號專家rose");// 設置按鈕上的文字
final Button button2 = new Button(shell, SWT.Activate);
button2.setBounds(500, 530, 200, 75); // 設置按鈕位置
button2.setFont(new Font(display, "宋體", 12, SWT.BOLD));
button2.setText("二號專家xiaoxiao");// 設置按鈕上的文字
final Button button3 = new Button(shell, SWT.Activate);
button3.setBounds(750, 530, 200, 75); // 設置按鈕位置
button3.setFont(new Font(display, "宋體", 12, SWT.BOLD));
button3.setText("三號專家yangguang");// 設置按鈕上的文字


button1.addSelectionListener(new SelectionAdapter()
{

public void widgetSelected(SelectionEvent e)
{
Doctor d1 = new Doctor("rose", 1);
Patient patient = new Patient();
// Queue<Patient> p1=m.get("xiaoxiao");
// d1.addPatient(patient);
rosePL.offer(patient);

patient.setpNum(rosePL.size());
patient.setFrontNum(rosePL.size() - 1);
// m.put(d1, p1);


if (rosePL.size() <= 30)
{
txt.setText("一號專家為您就診,\n" + "您現(xiàn)在排在" + rosePL.size()
+ "號,\n" + "前面人數(shù)為" + patient.getFrontNum());

} else
{
txt
.setText("一號專家為您就診" + "您現(xiàn)在排在" + rosePL.size() + "號"
+ "您前面已有" + patient.getFrontNum()
+ "人,\n您可以考慮其他專家");
}
//
}
});

button2.addSelectionListener(new SelectionAdapter()
{

public void widgetSelected(SelectionEvent e)
{
Doctor d2 = new Doctor("xiaoxiao", 1);
Patient patient = new Patient();
// d1.addPatient(patient);
xiaoPL.offer(patient);

patient.setpNum(xiaoPL.size());
patient.setFrontNum(xiaoPL.size() - 1);
// m.put(d2, p2);


if (xiaoPL.size() <= 30)
{
txt.setText("二號專家為您就診,\n" + "您現(xiàn)在排在" + rosePL.size()
+ "號,\n" + "前面人數(shù)為" + patient.getFrontNum());

} else
{
txt
.setText("二號專家為您就診,\n" + "您現(xiàn)在排在" + rosePL.size()
+ "號" + "您前面已有" + patient.getFrontNum()
+ "人,\n您可以考慮其他專家");
}
//
}
});

button3.addSelectionListener(new SelectionAdapter()
{

public void widgetSelected(SelectionEvent e)
{
Doctor d3 = new Doctor("yangguang", 1);
Patient patient = new Patient();
// d1.addPatient(patient);
yangPL.offer(patient);

patient.setpNum(yangPL.size());
patient.setFrontNum(yangPL.size() - 1);
// m.put(d3, yangPL);
// System.out.println(m.get(d3));


if (yangPL.size() <= 30)
{
txt.setText("三號專家為您就診,\n" + "您現(xiàn)在排在" + yangPL.size()
+ "號,\n" + "前面人數(shù)為" + patient.getFrontNum());

} else
{
txt.setText("您前面已有" + patient.getFrontNum()
+ "人,\n您可以考慮其他專家");
}
//
}
});

shell.layout();
shell.open();

while (!shell.isDisposed())
{
if (!display.readAndDispatch())
display.sleep();
}
}
}

醫(yī)生界面UI:
掛號時運行界面如下,點擊相應的專家會加到相應的專家中會是相應的專家為您就診:
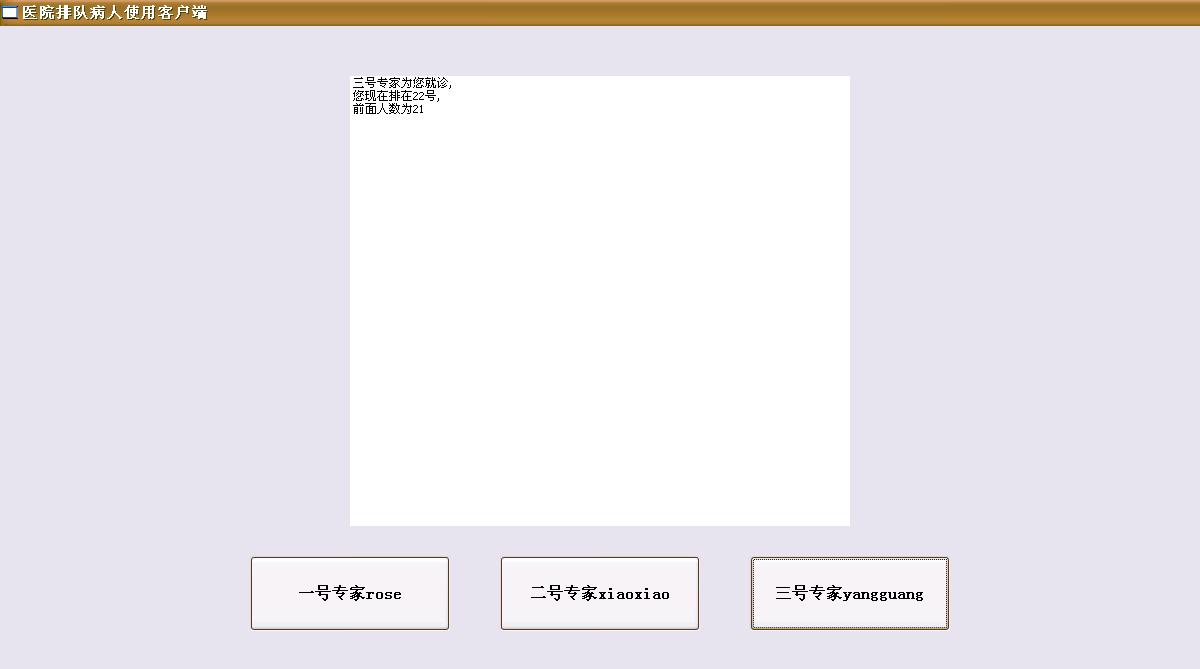
醫(yī)生叫號時運行界面如下,相應的專家點擊自己相應的名字可以出現(xiàn)自己相應的下一個病人的名字:
posted on 2010-11-02 16:51
迷人笑笑 閱讀(3463)
評論(1) 編輯 收藏