書中的一個例子,我也是想了半天了!!!有點難度!!!
/* 把多個文件的內容追加到一個文件中 */
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFSIZE 1024
#define SLEN 81
void append(FILE *source, FILE *dest);
int main(void)
{
FILE *fa, *fs; // fa指向追加的目的文件, fs指向源文件
int files = 0; // 追加文件的個數
char file_app[SLEN]; // 被追加文件的名稱
char file_src[SLEN]; // 源文件的名稱
puts("Enter name of destination file: ");
gets(file_app);
// "a":打開一個文本文件,可以寫入文件,向已有文件的尾部追加內容,如果
// 該文件不存在則先創建之
if((fa = fopen(file_app, "a")) == NULL)
{
fprintf(stderr, "Can't open %s\n", file_app);
exit(2);
}
/*
創建一個輸出緩沖區
NULL---函數會自動為自己分配一個緩沖區;
BUFSIZE---其大小為1024byte;
_IOFBF---完全緩沖(緩沖區滿的時候刷新)
*/
if(setvbuf(fa, NULL, _IOFBF, BUFSIZE) != 0)
{
fputs("Can't create output buffer\n", stderr);
exit(3);
}
puts("Enter name of first source file (empty line to quit): ");
while(gets(file_src) && file_src[0] != '\0')
{
if(strcmp(file_src, file_app) == 0)
fputs("Can't append file to itself\n", stderr);
else if((fs = fopen(file_src, "r")) == NULL)
fprintf(stderr, "Can't open %s\n", file_src);
else
{
/*
創建一個輸入緩沖區
NULL---函數會自動為自己分配一個緩沖區;
BUFSIZE---其大小為1024byte;
_IOFBF---完全緩沖(緩沖區滿的時候刷新)
*/
if(setvbuf(fs, NULL, _IOFBF, BUFSIZE) != 0)
{
fputs("Can't create input buffer\n", stderr);
continue;
}
append(fs, fa);
// 發生讀取錯誤
if(ferror(fs) != 0)
fprintf(stderr, "Error in reading file %s.\n", file_src);
// 發生寫入錯誤
if(ferror(fa) != 0)
fprintf(stderr, "Error in writing file %s.\n", file_app);
fclose(fs);
files++;
printf("File %s appended.\n", file_src);
puts("Next file(empty line to quit): ");
}
}
printf("Done. %d files appended.\n", files);
fclose(fa);
return 0;
}
void append(FILE *source, FILE *dest)
{
size_t bytes;
static char temp[BUFSIZE];
while((bytes = fread(temp, sizeof(char), BUFSIZE, source)) > 0)
fwrite(temp, sizeof(char), bytes, dest);
}
我的運行結果:
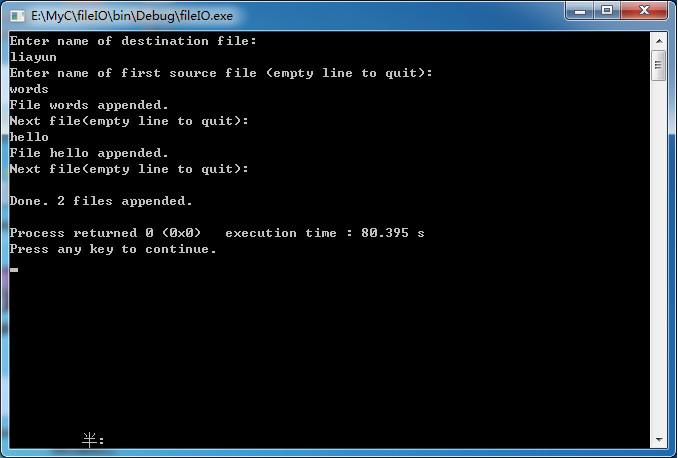
確實追加成功!!!
復習題1、下面的程序有什么問題?
int main(void)
{
int * fp;
int k;
fp = fopen("gelatin");
for(k = 0; k < 30; k++)
fputs(fp, "Nanette eats gelatin.");
fclose("gelatin");
return 0;
}
答:(參考課后答案)
因為程序中有文件定義,所以應該有#include<stdio.h>。應該把fp聲明為文件指針:FILE *fp;。函數fopen()需要一種模式:fopen("gelatin", "w");或"a"模式。fputs()函數中參數的次序應該反過來。為了清除起見,輸出字符串應該具有一個換行符,因為fputs()并不自動添加它。fclose()函數需要一個文件指針而不是文件名:fclose(fp);。下面是一個正確的版本:
#include <stdio.h>
int main(void)
{
FILE * fp;
int k;
fp = fopen("gelatin", "w");
for(k = 0; k < 30; k++)
fputs("Nanette eats gelatin.\n", fp);
fclose(fp);
return 0;
}
2、下面程序的作用是什么?(Macintosh用戶可以假設程序正確地使用了console.h和ccommand()函數。)
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
int main(int argc, char *argv[])
{
int ch;
FILE * fp;
if(argc < 2)
exit(2);
if((fp = fopen(argv[1], "r")) == NULL)
exit(1);
while((ch = getc(fp)) != EOF)
if(isdigit(ch))
putchar(ch);
fclose(fp);
return 0;
}
答:
如果可能的話,它會打開名字與第一個命令行參數相同的文件,并在屏幕上顯示文件中的每個數字字符。
3、假設在程序中有這樣一些語句:
#include <stdio.h>
FILE * fp1, * fp2;
char ch;
fp1 = fopen("terky", "r");
fp2 = fopen("jerky", "w");
并且,假設兩個文件都已被成功地打開了。為下面的函數調用提供缺少的參數:
a.ch = getc();
b.fprintf(, "%c\n",);
c.putc(,);
d.fclose(); /* 關閉terky文件 */
答:
a.ch = getc(fp1);
b.fprintf(fp2, "%c\n", ch);
c.putc(ch, fp2);
d.fclose(fp1); /* 關閉terky文件 */
4、編寫一段程序。它不讀取任何命令行參數或者讀取一個命令行參數。如果有一個參數,程序將它作為一個輸入文件名。如果沒有參數,使用標準輸入(stdin)作為輸入。假設輸入完全由浮點數組成。讓程序計算并且報告輸入數字的算數平均值。
答:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[])
{
int ch;
FILE * fp;
double n, sum = 0.0;
int ct = 0;
if(argc == 1)
fp = stdin;
else if(argc == 2)
{
if((fp = fopen(argv[1], "r")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[1]);
exit(EXIT_FAILURE);
}
}
else
{
fprintf(stderr, "Usage: %s [filename]\n", argv[0]);
exit(EXIT_FAILURE);
}
while(fscanf(fp, "%lf", &n) == 1)
{
sum += n;
++ct;
}
if(ct > 0)
printf("Average of %d values = %f\n", ct, sum / ct);
else
printf("No valid data.\n");
fclose(fp);
return 0;
}
5、編寫一段程序。它接受兩個命令行參數,第一個是一個字符,第二個是一個文件名。要求程序只打印文件中包含給定字符的哪些行。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[])
{
FILE * fp;
char input[1001];
if(argc < 3)
{
fprintf(stderr, "Usage: %s filename\n", argv[0]);
exit(EXIT_FAILURE);
}
if((fp = fopen(argv[2], "r")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[2]);
exit(EXIT_FAILURE);
}
while(fgets(input, 256, fp) != NULL)
{
if(strchr(input, argv[1][0]) != NULL)
fputs(input, stdout);
}
fclose(fp);
return 0;
}
6、對于二進制流而言,二進制文件和文本文件有什么區別?對于文本流呢?
答:(參考課后答案)
二進制文件和文本文件之間的不同在于這兩種文件格式對
系統的依賴性不同。二進制流和文本流之間的不同則包括在讀寫流時由程序執行的轉換(
二進制流不進行轉換,而文本流可能會轉換換行符和其他字符)。
7、下面兩者之間的區別是什么?
a.通過使用fprintf()和使用fwrite()保存8238201.
b.通過使用putc()和使用fwrite()保存字符S.
答:
a.使用fprintf()存儲8238201時,把它作為在7個字節中的7個字符來保存;而使用fwrite()來存儲時,使用該數值的二進制表示把它保存為一個4字節的整數。
b.沒有不同,每種情況下都保存為一個單字節的二進制碼。
8、下列語句的區別是什么?
printf("Hello, %s\n", name);
fprintf(stdout, "Hello, %s\n", name);
fprintf(stderr, "Hello, %s\n", name);
答:
第一個只是第二個的速記表示;第三個寫到標準錯誤上。通常標準錯誤被定向到與標準輸出同樣的位置,但是標準錯誤不受標準輸出重定向的影響。
9、以"a+"、"r+"和"w+"模式打開的文件都是可讀可寫的。哪種模式更適合用來改變文件中已有的內容?
答:"r+"模式使您可以在文件中的任何位置讀寫,所以它是最合適的。"a+"只允許您在文件的末尾添加內容,而"w+"給您提供一個空文件,它丟棄以前的文件內容。
編程練習1、
#include <stdio.h>
#include <stdlib.h>
#define SLEN 50
int main(void)
{
int ch;
FILE * fp;
char file[SLEN];
long count = 0;
puts("Enter the name of the file to be processed: ");
gets(file);
if((fp = fopen(file, "r")) == NULL)
{
fprintf(stderr, "Can't open %s\n", file);
exit(EXIT_FAILURE);
}
while((ch = getc(fp)) != EOF)
{
putc(ch, stdout);
count++;
}
fclose(fp);
printf("File %s has %ld characters\n", file, count);
return 0;
}
2、
#include <stdio.h>
#include <stdlib.h>
#define SLEN 50
int main(int argc, char *argv[])
{
FILE *in, *out;
int ch;
char file[SLEN];
if(argc < 3)
{
fprintf(stderr, "Usage: %s filename\n", argv[0]);
exit(EXIT_FAILURE);
}
if((in = fopen(argv[1], "rb")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[1]);
exit(EXIT_FAILURE);
}
if((out = fopen(argv[2], "wb")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[2]);
exit(EXIT_FAILURE);
}
// 復制
while((ch = getc(in)) != EOF)
putc(ch, out);
if(fclose(in) != 0 || fclose(out) != 0)
fprintf(stderr, "Error in closing files\n");
return 0;
}
3、
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define SLEN 50
int main(void)
{
FILE *in, *out;
int ch;
char file_source[SLEN], file_target[SLEN];
printf("Enter the source file name and the output file name: \n");
scanf("%s %s", file_source, file_target);
if((in = fopen(file_source, "r")) == NULL)
{
fprintf(stderr, "Can't open %s\n", file_source);
exit(EXIT_FAILURE);
}
if((out = fopen(file_target, "w")) == NULL)
{
fprintf(stderr, "Can't open %s\n", file_target);
exit(EXIT_FAILURE);
}
// 復制
while((ch = getc(in)) != EOF)
putc(toupper(ch), out);
if(fclose(in) != 0 || fclose(out) != 0)
fprintf(stderr, "Error in closing files\n");
return 0;
}
4、
#include <stdio.h>
#include <stdlib.h>
#define SLEN 50
int main(int argc, char *argv[])
{
FILE *fp;
int ch;
int i;
// 命令行參數只有程序名時
if(argc < 2)
{
printf("Usage: %s filename\n", argv[0]);
exit(EXIT_FAILURE);
}
// 命令行參數中有文件名時
for(i = 1; i < argc; i++)
{
if((fp = fopen(argv[i], "r")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[i]);
exit(EXIT_FAILURE);
}
printf("-------------------------file %s content-------------------------\n", argv[i]);
while((ch = getc(fp)) != EOF)
putc(ch, stdout);
putchar('\n');
if(fclose(fp) != 0)
fprintf(stderr, "Error in closing files\n");
}
return 0;
}
5、
/* 把多個文件的內容追加到一個文件中 */
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFSIZE 1024
#define SLEN 81
void append(FILE *source, FILE *dest);
int main(int argc, char *argv[])
{
FILE *fa, *fs; // fa指向追加的目的文件, fs指向源文件
int files = 0; // 追加文件的個數
int i = 2;
//char file_app[SLEN]; // 被追加文件的名稱
//char file_src[SLEN]; // 源文件的名稱
//puts("Enter name of destination file: ");
//gets(file_app);
// "a":打開一個文本文件,可以寫入文件,向已有文件的尾部追加內容,如果
// 該文件不存在則先創建之
if((fa = fopen(argv[1], "a")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[1]);
exit(2);
}
/*
創建一個輸出緩沖區
NULL---函數會自動為自己分配一個緩沖區;
BUFSIZE---其大小為1024byte;
_IOFBF---完全緩沖(緩沖區滿的時候刷新)
*/
if(setvbuf(fa, NULL, _IOFBF, BUFSIZE) != 0)
{
fputs("Can't create output buffer\n", stderr);
exit(3);
}
//puts("Enter name of first source file (empty line to quit): ");
while(i < argc)
{
if(strcmp(argv[i], argv[1]) == 0)
fputs("Can't append file to itself\n", stderr);
else if((fs = fopen(argv[i], "r")) == NULL)
fprintf(stderr, "Can't open %s\n", argv[i]);
else
{
/*
創建一個輸入緩沖區
NULL---函數會自動為自己分配一個緩沖區;
BUFSIZE---其大小為1024byte;
_IOFBF---完全緩沖(緩沖區滿的時候刷新)
*/
if(setvbuf(fs, NULL, _IOFBF, BUFSIZE) != 0)
{
fputs("Can't create input buffer\n", stderr);
continue;
}
append(fs, fa);
// 發生讀取錯誤
if(ferror(fs) != 0)
fprintf(stderr, "Error in reading file %s.\n", argv[i]);
// 發生寫入錯誤
if(ferror(fa) != 0)
fprintf(stderr, "Error in writing file %s.\n", argv[1]);
fclose(fs);
files++;
printf("File %s appended.\n", argv[i]);
i++;
}
}
printf("Done. %d files appended.\n", files);
fclose(fa);
return 0;
}
void append(FILE *source, FILE *dest)
{
size_t bytes;
static char temp[BUFSIZE];
while((bytes = fread(temp, sizeof(char), BUFSIZE, source)) > 0)
fwrite(temp, sizeof(char), bytes, dest);
}
我覺得沒必要用循環吧!使用命令行參數不像交互式界面一樣可以用循環表達的那么清楚, 這是我的第二次修改:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFSIZE 1024
#define SLEN 81
void append(FILE *source, FILE *dest);
int main(int argc, char *argv[])
{
FILE *fa, *fs;
int files = 0;
if(argc != 3)
{
printf("Usage: %s filename filename\n", argv[0]);
exit(EXIT_FAILURE);
}
if((fa = fopen(argv[1], "a")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[1]);
exit(EXIT_FAILURE);
}
if(setvbuf(fa, NULL, _IOFBF, BUFSIZE) != 0)
{
fputs("Can't create output buffer\n", stderr);
exit(EXIT_FAILURE);
}
if(strcmp(argv[1], argv[2]) == 0)
{
fputs("Can't append file to itself\n", stderr);
exit(EXIT_FAILURE);
}
else if((fs = fopen(argv[2], "r")) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[2]);
exit(EXIT_FAILURE);
}
else
{
if(setvbuf(fs, NULL, _IOFBF, BUFSIZE) != 0)
{
fputs("Can't create input buffer\n", stderr);
exit(EXIT_FAILURE);
}
append(fs, fa);
if(ferror(fs) != 0)
fprintf(stderr, "Error in reading file %s.\n", argv[2]);
if(ferror(fa) != 0)
fprintf(stderr, "Error in writing file %s.\n", argv[1]);
fclose(fs);
files++;
printf("File %s appended.\n", argv[2]);
}
printf("Done. %d files appended.\n", files);
fclose(fa);
return 0;
}
void append(FILE *source, FILE *dest)
{
size_t bytes;
static char temp[BUFSIZE];
while((bytes = fread(temp, sizeof(char), BUFSIZE, source)) > 0)
fwrite(temp, sizeof(char), bytes, dest);
}
6、
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LEN 40
int main(void)
{
FILE *in, *out;
int ch;
char name1[LEN];
char name2[LEN];
int count = 0;
puts("Enter the name of the file to be processed: ");
gets(name1);
if((in = fopen(name1, "r")) == NULL)
{
fprintf(stderr, "I couldn't open the file \"%s\"\n", name1);
exit(2);
}
strcpy(name2, name1);
strcat(name2, ".red");
if((out = fopen(name2, "w")) == NULL)
{
fprintf(stderr, "Can't create output file.\n");
exit(3);
}
while((ch = getc(in)) != EOF)
if(count++ % 3 == 0)
putc(ch, out); // 打印每3個字符中的1個
if(fclose(in) != 0 || fclose(out) != 0)
fprintf(stderr, "Error in closing files.\n");
return 0;
}
聲明兩個字符數組,沒必要!
經過第二次修改如下:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LEN 40
int main(void)
{
FILE *in, *out;
int ch;
char name[LEN];
int count = 0;
puts("請您輸入要讀取的文件名:");
gets(name);
if((in = fopen(name, "r")) == NULL)
{
fprintf(stderr, "I couldn't open the file \"%s\"\n", name);
exit(EXIT_FAILURE);
}
strcat(name, ".red");
if((out = fopen(name, "w")) == NULL)
{
fprintf(stderr, "Can't create output file.\n");
exit(EXIT_FAILURE);
}
while((ch = getc(in)) != EOF)
if(count++ % 3 == 0)
putc(ch, out);
if(fclose(in) != 0 || fclose(out) != 0)
fprintf(stderr, "Error in closing files\n");
return 0;
}
7、(參考
http://xiongyi85.blog.51cto.com)
//pe13-7a
#include <stdio.h>
#include <stdlib.h>
#define SIZE 256
int main(int argc, char *argv[])
{
FILE *fp1, *fp2;
char ch1, ch2;
if(argc < 3)
{
fprintf(stderr, "Usage: %s filename\n", argv[0]);
exit(EXIT_FAILURE);
}
if((fp1 = fopen(argv[1], "r")) == NULL)
{
fprintf(stderr, "I couldn't open the file \"%s\"\n", argv[1]);
exit(1);
}
if((fp2 = fopen(argv[2], "r")) == NULL)
{
fprintf(stderr, "I couldn't open the file \"%s\"\n", argv[2]);
exit(2);
}
// 不應該用fgets()/fputs()函數
ch1 = fgetc(fp1);
ch2 = fgetc(fp2);
while(ch1 != EOF || ch2 != EOF)
{
if(ch1 == EOF || ch2 == EOF)
putchar('\n');
while(ch1 != EOF && ch1 != '\n')
{
putchar(ch1);
ch1 = fgetc(fp1);
}
if(ch1 != EOF)
{
putchar('\n');
ch1 = fgetc(fp1);
}
while(ch2 != EOF && ch2 != '\n')
{
putchar(ch2);
ch2 = fgetc(fp2);
}
if(ch2 != EOF)
{
putchar('\n');
ch2 = fgetc(fp2);
}
}
/*while(fgets(line1, SIZE, fp1) != NULL || fgets(line2, SIZE, fp2) != NULL)
{
while(*line1 != '\n')
putchar(*line1++);
putchar('\n');
while(*line2 != '\n')
putchar(*line2++);
}*/
if(fclose(fp1) != 0 || fclose(fp2) != 0)
fprintf(stderr, "Error in closing files.\n");
return 0;
}
//pe13-7b
#include <stdio.h>
#include <stdlib.h>
#define SIZE 256
int main(int argc, char *argv[])
{
FILE *fp1, *fp2;
char ch1, ch2;
if(argc < 3)
{
fprintf(stderr, "Usage: %s filename\n", argv[0]);
exit(EXIT_FAILURE);
}
if((fp1 = fopen(argv[1], "r")) == NULL)
{
fprintf(stderr, "I couldn't open the file \"%s\"\n", argv[1]);
exit(1);
}
if((fp2 = fopen(argv[2], "r")) == NULL)
{
fprintf(stderr, "I couldn't open the file \"%s\"\n", argv[2]);
exit(2);
}
// 不應該用fgets()/fputs()函數
ch1 = fgetc(fp1);
ch2 = fgetc(fp2);
while(ch1 != EOF || ch2 != EOF)
{
if(ch1 == EOF || ch2 == EOF)
putchar('\n');
while(ch1 != EOF && ch1 != '\n')
{
putchar(ch1);
ch1 = fgetc(fp1);
}
// 不打印換行符,改為打印一個空格
if(ch1 != EOF)
{
putchar(' ');
ch1 = fgetc(fp1);
}
while(ch2 != EOF && ch2 != '\n')
{
putchar(ch2);
ch2 = fgetc(fp2);
}
if(ch2 != EOF)
{
putchar('\n');
ch2 = fgetc(fp2);
}
}
if(fclose(fp1) != 0 || fclose(fp2) != 0)
fprintf(stderr, "Error in closing files.\n");
return 0;
}
8、
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[])
{
FILE *fp;
char ch;
int i, count = 0;
if(argc == 2)
fp = stdin;
else
{
for(i = 2; i < argc; i++)
{
if((fp = fopen(argv[i], "r")) == NULL)
{
fprintf(stderr, "I couldn't open the file \"%s\"\n", argv[i]);
exit(1);
}
while((ch = fgetc(fp)) != EOF)
if(argv[1][0] == ch)
count++;
if(fclose(fp) != 0)
fprintf(stderr, "Error in closing files.\n");
printf("The number of characters %c in file %s: %d\n", argv[1][0], argv[i], count);
}
}
return 0;
}
沒有fgetc()函數,只有fgets()函數,注意區分getc()、fgets()函數!!!
經第二次修改如下:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LEN 40
int main(int argc, char *argv[])
{
int ch;
int count = 0;
FILE *fp;
if(argc < 2)
{
fprintf(stderr, "Usage: %s char(or filename): \n", argv[0]);
exit(EXIT_FAILURE);
}
if(argc == 2)
{
printf("請您輸入一些字符:\n");
while((ch = getchar()) != '\n')
{
if(ch == argv[1][0])
count++;
}
printf("字符%c出現的次數為:%d\n", argv[1][0], count);
}
else
{
for(int i = 2; i < argc; i++)
{
count = 0;
if((fp = fopen(argv[i], "r")) == NULL)
{
fprintf(stderr, "Can't open the file %s.\n", argv[i]);
exit(EXIT_FAILURE);
}
while((ch = getc(fp)) != EOF)
{
if(ch == argv[1][0])
count++;
}
if(fclose(fp) != 0)
printf("Error in closing files.\n");
printf("字符%c在文件%s中出現的次數為:%d\n", argv[1][0], argv[i], count);
}
}
return 0;
}
9、(媽的,理解錯題意了,我還以為是在一個已經存在的文件繼續追加單詞,我說怎么列表順序不是從1開始的,浪費太多時間了!參考,
http://ptgmedia.pearsoncmg.com/images/9780321928429/downloads/9780321928429_ProgrammingExerciseAnswers_Selected.pdf)注意審題!!!#include <stdio.h>
#include <stdlib.h>
#define MAX 40
int main(void)
{
FILE *fp;
char words[MAX];
int word_count = 0;
if((fp = fopen("wordy", "a+")) == NULL)
{
fprintf(stdout, "Can't open \"wordy\" file.\n");
exit(1);
}
/* 確定文件當前行數 */
rewind(fp); // 使程序回到文件開始處
while((fgets(words, MAX, fp)) != NULL)
word_count++;
rewind(fp); // 使程序回到文件開始處
puts("Enter words to add to the file; press the Enter");
puts("key at the beginning of a line to terminate.");
while(gets(words) != NULL && words[0] != '\0')
fprintf(fp, "%d:%s\n", ++word_count, words);
puts("File contents: ");
rewind(fp);
while(fgets(words, MAX, fp) != NULL)
fputs(words, stdout);
if(fclose(fp) != 0)
fprintf(stderr, "Error closing file\n");
return 0;
}
10、(做的想吐血,我真是受不了了!!!為什么我寫的就不對呢?借鑒
http://xiongyi85.blog.51cto.com做法)
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX 40
int main(void)
{
FILE *fp;
char file[MAX];
long location, last;
char ch;
puts("Enter the name of the file to be processed: ");
gets(file);
// 以文本模式打開文件
if((fp = fopen(file, "r")) == NULL)
{
printf("reverse can't open %s\n", file);
exit(EXIT_FAILURE);
}
fseek(fp, 0L, SEEK_END);
last = ftell(fp);
printf("Please enter a file location (q to quit): \n");
while(scanf("%ld", &location) == 1)
{
if(location < last && location > 0)
{
fseek(fp, location - 1, SEEK_SET); // 找到文件的第location個字節處
while((ch = fgetc(fp)) != '\n') // 適用范圍:只是對于第一行有效,而不能擴展至任意行
putchar(ch);
putchar('\n');
}
else
printf("out of range!\n");
printf("Please enter a file location (q to quit): \n");
}
// 為什么不能像下面這樣寫呢???
/*fseek(fp, location, SEEK_SET); // 找到文件的第location個字節處
for(count = 0L; count < last; count++)
{
fseek(fp, count, SEEK_CUR);
ch = getc(fp);
if(ch != '\n')
putchar(ch);
}*/
if(fclose(fp) != 0)
fprintf(stderr, "Error closing file\n");
return 0;
}
11、
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 40
int main(int argc, char *argv[])
{
FILE *fp;
char line[SIZE];
if(argc < 3)
{
fprintf(stderr, "Usage: %s string filename\n", argv[0]);
exit(EXIT_FAILURE);
}
if((fp = fopen(argv[2], "r")) == NULL)
{
fprintf(stderr, "Can't open the file \"%s\"\n", argv[2]);
exit(EXIT_FAILURE);
}
while(fgets(line, SIZE, fp) != NULL)
if(strstr(line, argv[1]) != NULL)
fputs(line, stdout);
if(fclose(fp) != 0)
fprintf(stderr, "Error closing file\n");
return 0;
}
12、(實在做不出來,遂參考
http://ptgmedia.pearsoncmg.com/images/9780321928429/downloads/9780321928429_ProgrammingExerciseAnswers_Selected.pdf)#include <stdio.h>
#include <stdlib.h>
#define ROWS 20
#define COLS 30
#define LEVELS 10
const char trans[LEVELS + 1] = " .':~*=&%@";
// 初始化字符數組
void init(char arr[][COLS], char ch); // arr指向一個包含30個字符的數組
void makePic(char pic[][COLS], int data[][COLS], int row);
int main(void)
{
int row, col;
FILE *fp;
char ch[ROWS][COLS]; // 創建一個20*30的字符數組
int image[ROWS][COLS];
if((fp = fopen("wordy", "r")) == NULL)
{
fprintf(stderr, "Can't open the file \"wordy\"\n");
exit(EXIT_FAILURE);
}
// 將文件的內容讀入到一個20*30的int數組中
for(row = 0; row < ROWS; row++)
for(col = 0; col < COLS; col++)
fscanf(fp, "%d", &image[row][col]); // scanf()函數忽略空格和換行符
// 如果發生讀取錯誤
if(ferror(fp) != 0)
{
fprintf(stderr, "Error in reading file wordy.\n");
exit(EXIT_FAILURE);
}
makePic(ch, image, ROWS);
// 向屏幕輸出圖片
for(row = 0; row < ROWS; row++)
{
for(col = 0; col < COLS; col++)
putchar(ch[row][col]);
putchar('\n');
}
if(fclose(fp) != 0)
fprintf(stderr, "Error closing file\n");
return 0;
}
void init(char arr[][COLS], char ch)
{
int r, c;
for(r = 0; r < ROWS; r++)
for(c = 0; c < COLS; c++)
arr[r][c] = ch;
}
//將data數組的值轉換成trans中的符號后錄入pic
void makePic(char pic[][COLS], int data[][COLS], int row)
{
int r, c;
for(r = 0; r < row; r++)
for(c = 0; c < COLS; c++)
pic[r][c] = trans[data[r][c]]; // 驚呆小伙伴了!!!
}
我的文件:
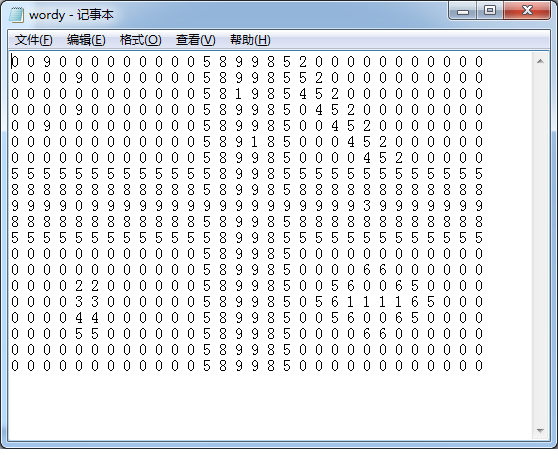
運行結果:
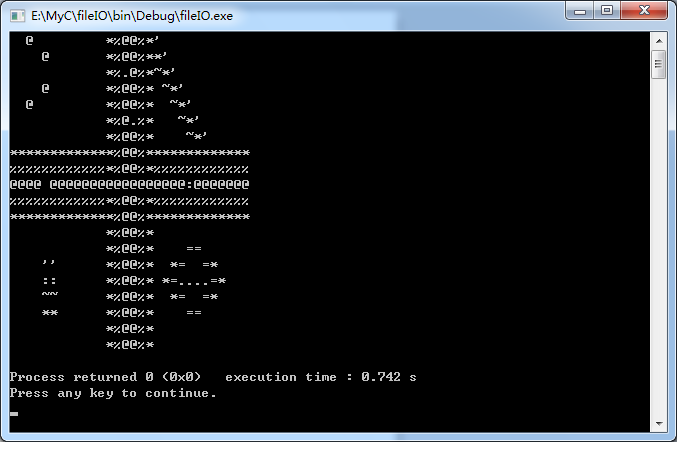
13、(參考
csdn---vs9841前輩的做法,但做的方法太笨了,應該還有簡便方法的,不用考慮這9種情況的!因為太繁瑣了!)
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <stdbool.h>
#define ROWS 20
#define COLS 30
#define LEVELS 10
const char trans[LEVELS + 1] = " .':~*=&%@";
// 初始化字符數組
void init(char arr[][COLS], char ch); // arr指向一個包含30個字符的數組
// 消除尖峰脈沖
void eliminate(int row, int col, int data[][COLS]);
// 判斷該值與它周圍每個值的差都是否大于1
bool check(int n, int te[n]);
void makePic(char pic[][COLS], int data[][COLS], int row);
int main(void)
{
int row, col;
FILE *fp;
char ch[ROWS][COLS]; // 創建一個20*30的字符數組
int image[ROWS][COLS];
if((fp = fopen("wordy", "r")) == NULL)
{
fprintf(stderr, "Can't open the file \"wordy\"\n");
exit(EXIT_FAILURE);
}
// 將文件的內容讀入到一個20*30的int數組中
for(row = 0; row < ROWS; row++)
for(col = 0; col < COLS; col++)
fscanf(fp, "%d", &image[row][col]); // scanf()函數忽略空格和換行符
// 如果發生讀取錯誤
if(ferror(fp) != 0)
{
fprintf(stderr, "Error in reading file wordy.\n");
exit(EXIT_FAILURE);
}
eliminate(ROWS, COLS, image);
makePic(ch, image, ROWS);
// 向屏幕輸出圖片
for(row = 0; row < ROWS; row++)
{
for(col = 0; col < COLS; col++)
putchar(ch[row][col]);
putchar('\n');
}
if(fclose(fp) != 0)
fprintf(stderr, "Error closing file\n");
return 0;
}
void init(char arr[][COLS], char ch)
{
int r, c;
for(r = 0; r < ROWS; r++)
for(c = 0; c < COLS; c++)
arr[r][c] = ch;
}
void eliminate(int row, int col, int data[][COLS])
{
int r, c;
int temp[4] = {[1] = 0}; // 存放與上下左右相減的絕對值
for(r = 0; r < row; r++)
for(c = 0; c < col; c++)
{
if(r == 0)
{
if(c == 0) // 矩陣的左上角
{
temp[0] = abs(data[r][c] - data[r+1][c]);
temp[1] = abs(data[r][c] - data[r][c+1]);
if(check(2, temp))
data[r][c] = (data[r+1][c] + data[r][c+1]) / 2;
}
else if(c == col - 1) // 矩陣的右上角
{
temp[0] = abs(data[r][c] - data[r][c-1]);
temp[1] = abs(data[r][c] - data[r+1][c]);
if(check(2, temp))
data[r][c] = (data[r][c-1] + data[r+1][c]) / 2;
}
else // 矩陣的上邊
{
temp[0] = abs(data[r][c] - data[r][c-1]);
temp[1] = abs(data[r][c] - data[r][c+1]);
temp[2] = abs(data[r][c] - data[r+1][c]);
if(check(3, temp))
data[r][c] = (data[r][c-1] + data[r][c+1] + data[r+1][c]) / 3;
}
}
else if(r == row - 1)
{
if(c == 0) // 矩陣的左下角
{
temp[0] = abs(data[r][c] - data[r-1][c]);
temp[1] = abs(data[r][c] - data[r][c+1]);
if(check(2, temp))
data[r][c] = (data[r-1][c] + data[r][c+1]) / 2;
}
else if(c == col - 1) // 矩陣的右下角
{
temp[0] = abs(data[r][c] - data[r-1][c]);
temp[1] = abs(data[r][c] - data[r][c-1]);
if(check(2, temp))
data[r][c] = (data[r-1][c] + data[r][c-1]) / 2;
}
else // 矩陣的下邊
{
temp[0] = abs(data[r][c] - data[r-1][c]);
temp[1] = abs(data[r][c] - data[r][c-1]);
temp[2] = abs(data[r][c] - data[r][c+1]);
if(check(3, temp))
data[r][c] = (data[r-1][c] + data[r][c-1] + data[r][c+1]) / 3;
}
}
else if(c == 0) // 矩陣的左邊
{
temp[0] = abs(data[r][c] - data[r-1][c]);
temp[1] = abs(data[r][c] - data[r][c+1]);
temp[2] = abs(data[r][c] - data[r+1][c]);
if(check(3, temp))
data[r][c] = (data[r-1][c] + data[r][c+1] + data[r+1][c]) / 3;
}
else if(c == col - 1) // 矩陣的右邊
{
temp[0] = abs(data[r][c] - data[r-1][c]);
temp[1] = abs(data[r][c] - data[r][c-1]);
temp[2] = abs(data[r][c] - data[r+1][c]);
if(check(3, temp))
data[r][c] = (data[r-1][c] + data[r][c-1] + data[r+1][c]) / 3;
}
else // 矩陣的內部
{
temp[0] = abs(data[r][c] - data[r-1][c]);
temp[1] = abs(data[r][c] - data[r][c-1]);
temp[2] = abs(data[r][c] - data[r+1][c]);
temp[3] = abs(data[r][c] - data[r][c+1]);
if(check(4, temp))
data[r][c] = (data[r-1][c] + data[r][c-1] + data[r+1][c] + data[r][c+1]) / 4;
}
}
}
bool check(int n, int te[])
{
int i;
for(i = 0; i < n; i++)
if(te[i] <= 1)
return false;
return true;
}
//將data數組的值轉換成trans中的符號后錄入pic
void makePic(char pic[][COLS], int data[][COLS], int row)
{
int r, c;
for(r = 0; r < row; r++)
for(c = 0; c < COLS; c++)
pic[r][c] = trans[data[r][c]]; // 驚呆小伙伴了!!!
}
我的文件:
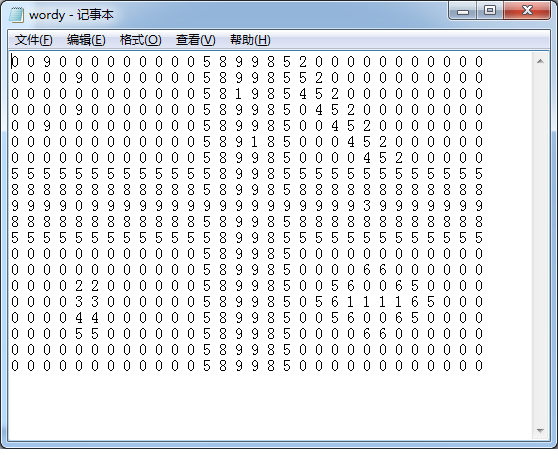
運行結果為:
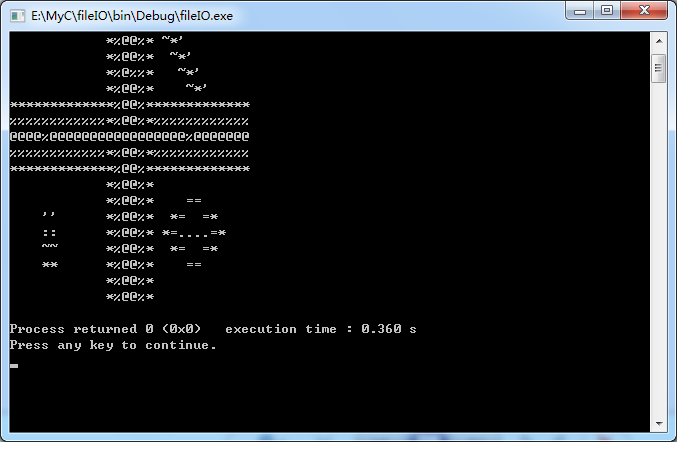
至此,終于完成這章編程練習,真是不容易!!!