Most developers have heard of, and possibly used, scripting languages
such as Ruby, JavaScript, and Python. These dynamic languages are
enjoying a resurgence in popularity, largely because of their
flexibility and simplicity, and the productivity gains they promise.
Java 6 comes with built-in support for scripting languages.
You can embed scripts in various scripting languages into your Java
applications, passing parameters, evaluating expressions, and
retrieving results. And you can do it all pretty seamlessly.
First of all, you obtain a new ScriptEngine object from a ScriptEngineManager, as shown here:
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("js");
Each scripting language has its own unique identifier. The "js" here means you're dealing with JavaScript.
Now you can start having some fun. Interacting with a script is easy
and intuitive. You can assign scripting variables using the put() method and evaluate the script using the eval()
method,. which returns the most recently evaluated expression processed
by the script. And that pretty much covers the essentials. Here's an
example that puts it all together:
engine.put("cost", 1000);
String decision = (String) engine.eval(
"if ( cost >= 100){ " +
"decision = 'Ask the boss'; " +
"} else {" +
"decision = 'Buy it'; " +
"}");
assert ("Ask the boss".equals(decision));
You can do more than just pass variables to your scripts— you can
also invoke Java classes from within your scripts. Using the
importPackage() function enables you to import Java packages, as shown
here:
engine.eval("importPackage(java.util); " +
"today = new Date(); " +
"print('Today is ' + today);");
Another cool feature is the Invocable
interface, which lets you invoke a function by name within a script.
This lets you write libraries in scripting languages, which you can use
by calling key functions from your Java application. You just pass the
name of the function you want to call, an array of Objects for the
parameters, and you're done! Here's an example:
engine.eval("function calculateInsurancePremium(age) {...}");
Invocable invocable = (Invocable) engine;
Object result = invocable.invokeFunction("calculateInsurancePremium",
new Object[] {37});
You actually can do a fair bit more than what I've shown here. For
example, you can pass a Reader object to the eval() method, which makes
it easy to store scripts in external files, or bind several Java
objects to JavaScript variables using a Map-like Binding object. You
can also compile some scripting languages to speed up processing. But
you probably get the idea that the integration with Java is smooth and
well thought-out.
posted @
2007-03-07 21:49 wqwqwqwqwq 閱讀(468) |
評論 (0) |
編輯 收藏
剛剛結束了IBM的電面,一個GG和JJ,問的主要問題都放在我的項目上了,尤其是Netbeans的插件。由于我申請的實習生,所以在之中也問了很多實習時間的問題,感覺IBM問問題很不含糊,甚至要問出幾月幾號......這一點可以說明IBM是一個很值得人去的地方。英文問題我感覺我答得不是很好,先問得IBM的文化之類的,之后居然問得IBM person慚愧啊 本來還知道幾個的,一緊張都忘了。在角色兌換時,我問得我的表現,和職業生涯建議,好小子,他們居然還反問我職業生涯,說了一通......整個電面是從4:10-4:45,最后GG說面試表現還好,只是我能實習的時間比他們要求的時間要少,唉~~ 我的系主任啊,等待結果中....希望自己能去IBM體驗一下藍色巨人的風范吧。
posted @
2007-03-02 17:05 wqwqwqwqwq 閱讀(803) |
評論 (0) |
編輯 收藏
一會4:30要參加北京IBM研究院的一個電面,快到時間了,不免心里稍有些緊張,不知道先前積累的一點英語一會是否會奏效,呵呵,,,,不過,無論如何我會盡可能抓住這次來之不易的機會的。
posted @
2007-03-02 14:44 wqwqwqwqwq 閱讀(533) |
評論 (0) |
編輯 收藏
摘要: This post had been writen long before,...but
閱讀全文
posted @
2007-02-15 23:36 wqwqwqwqwq 閱讀(470) |
評論 (1) |
編輯 收藏
摘要: Java SE 6.0(代號Mustang,野馬)已經發布,詳情請見
野馬奔騰而出,Java SE 6 正式版發布
,它給我們帶來了哪些新的特性了。
首先,我們看看JDK 6.0包含了大量的JSR,分為四組,分別為:
在簡化開發方面:
199: Compiler API...
閱讀全文
posted @
2007-02-08 11:18 wqwqwqwqwq 閱讀(2037) |
評論 (1) |
編輯 收藏
前幾天好不容易下到了JDK6mustang,今天恰好有時間升級了一下Netbeans默認的JDK版本。這里簡單的說明一下升級的方法。如果我
們不修改Netbeans的屬性,需要在JavaPlatform
manager中加入另一版本的類庫。新建工程后如果要修改類庫,還需要修改項目的類庫屬性,現在通過修改默認的JDK類庫,便可方便很多,更不需要重新
安裝NB。
我的NB裝在D盤中,可以在該路徑找到文件D:\Netbeans-5.5\etc\Netbeans.conf,我們將原有的默認類庫netbeans_jdkhome="D:\Java\jdk1.5.0_07"修改為
netbeans_jdkhome="D:\Java\jdk1.6.0"便輕松的完成了升級,當然在tools-〉JavaPlatform
manager〉中當然也可以將我們慣用的D:\Java\jdk1.5.0_07加入為可選用類庫。
posted @
2007-01-30 23:01 wqwqwqwqwq 閱讀(477) |
評論 (0) |
編輯 收藏
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312">
<title>拖拽Demo</title>
<style type="text/CSS">
body
{
margin:0px;
}
#aim
{
position:absolute;
width:200px;
height:30px;
border:1px solid #666666;
background-color:#FFCCCC;
}
#sourceLayer, #cloneLayer
{
position:absolute;
width:300px;
height:50px;
border:1px solid #666666;
background-color:#CCCCCC;
cursor:move;
}
.docked
{
display:none;
filter:alpha(opacity=100);
}
.actived
{
display:block;
filter:alpha(opacity=70);
}
</style>
</head>
<body >
<div id="aim">locate</div>
<div id="sourceLayer" unselectable="off"><img src="http://www.baidu.com/img/logo.gif" alt="Drag Demo">Source of the demo</div>
<div id="cloneLayer" class="docked" unselectable="off"></div>
<script type="text/javascript" language="javascript">
var aim;
var sourceLayer;
var cloneLayer;
var aimX;
var aimY;
var orgnX;
var orgnY;
var draging = false;
var offsetX = 0;
var offsetY = 0;
var back;
var thisX ;
var thisY ;
var time ;
var stepX ;
var stepY ;
function getLayer(inAim,inSource,inClone)
{
aim = document.getElementById(inAim);
sourceLayer = document.getElementById(inSource);
cloneLayer = document.getElementById(inClone);
}
function initDrag(initAimX,initAimY,initOrgnX,initOrgnY)
{
aimX = initAimX;
aimY = initAimY;
orgnX = initOrgnX;
orgnY = initOrgnY;
aim.style.pixelLeft = aimX;
aim.style.pixelTop = aimY;
sourceLayer.style.pixelLeft = orgnX;
sourceLayer.style.pixelTop = orgnY;
cloneLayer.style.pixelLeft = orgnX;
cloneLayer.style.pixelTop = orgnY;
}
function BeforeDrag()
{
if (event.button != 1)
{
return;
}
cloneLayer.innerHTML = sourceLayer.innerHTML; 容
offsetX = document.body.scrollLeft + event.clientX - sourceLayer.style.pixelLeft;
offsetY = document.body.scrollTop + event.clientY - sourceLayer.style.pixelTop;
cloneLayer.className = "actived";
draging = true;
}
function OnDrag()
{
if(!draging)
{
return;
}
event.returnValue = false;
cloneLayer.style.pixelLeft = document.body.scrollLeft + event.clientX - offsetX;
cloneLayer.style.pixelTop = document.body.scrollTop + event.clientY - offsetY;
}
function EndDrag()
{
if (event.button != 1)
{
return;
}
draging = false;
if (event.clientX >= aim.style.pixelLeft && event.clientX <= (aim.style.pixelLeft + aim.offsetWidth) &&
event.clientY >= aim.style.pixelTop && event.clientY <= (aim.style.pixelTop + aim.offsetHeight))
{
sourceLayer.style.pixelLeft = aim.style.pixelLeft;
sourceLayer.style.pixelTop = aim.style.pixelTop;
cloneLayer.className = "docked";
}
else
{
thisX = cloneLayer.style.pixelLeft;
thisY = cloneLayer.style.pixelTop;
offSetX = Math.abs(thisX - orgnX);
offSetY = Math.abs(thisY - orgnY);
time = 500;
stepX = Math.floor((offSetX/time)*20);
stepY = Math.floor((offSetY/time)*20);
if(stepX == 0)
stepX = 2;
if(stepY == 0)
stepY = 2;
moveStart();
}
}
function moveStart()
{
back = setInterval("MoveLayer();",15);
}
function MoveLayer()
{
if(cloneLayer.style.pixelLeft <= orgnX && cloneLayer.style.pixelTop <= orgnY)
{
cloneLayer.style.pixelLeft += stepX;
cloneLayer.style.pixelTop += stepY;
if(cloneLayer.style.pixelLeft > orgnX)
{
stepX = 1;
}
if(cloneLayer.style.pixelTop > orgnY)
{
stepY = 1;
}
//if the coordinate of X Y are same
if(cloneLayer.style.pixelLeft == orgnX)
{
stepX = 0;
}
if(cloneLayer.style.pixelTop == orgnY)
{
stepY = 0;
}
if(cloneLayer.style.pixelLeft == orgnX && cloneLayer.style.pixelTop == orgnY)
{
EndMove();
}
}
//locate to the downleft of the object
else if(cloneLayer.style.pixelLeft <= orgnX && cloneLayer.style.pixelTop >= orgnY)
{
cloneLayer.style.pixelLeft += stepX;
cloneLayer.style.pixelTop -= stepY;
if(cloneLayer.style.pixelLeft > orgnX)
{
stepX = 1;
}
if(cloneLayer.style.pixelTop < orgnY)
{
stepY = 1;
}
if(cloneLayer.style.pixelLeft == orgnX)
{
stepX = 0;
}
if(cloneLayer.style.pixelTop == orgnY)
{
stepY = 0;
}
if(cloneLayer.style.pixelLeft == orgnX && cloneLayer.style.pixelTop == orgnY)
{
EndMove();
}
}
else if(cloneLayer.style.pixelLeft >= orgnX && cloneLayer.style.pixelTop <= orgnY)
{
cloneLayer.style.pixelLeft -= stepX;
cloneLayer.style.pixelTop += stepY;
if(cloneLayer.style.pixelLeft < orgnX)
{
stepX = 1;
}
if(cloneLayer.style.pixelTop > orgnY)
{
stepY = 1;
}
if(cloneLayer.style.pixelLeft == orgnX)
{
stepX = 0;
}
if(cloneLayer.style.pixelTop == orgnY)
{
stepY = 0;
}
if(cloneLayer.style.pixelLeft == orgnX && cloneLayer.style.pixelTop == orgnY)
{
EndMove();
}
}
//locate to the right of the object
else if(cloneLayer.style.pixelLeft >= orgnX && cloneLayer.style.pixelTop >= orgnY)
{
cloneLayer.style.pixelLeft -= stepX;
cloneLayer.style.pixelTop -= stepY;
if(cloneLayer.style.pixelLeft < orgnX)
{
stepX = 1;
}
if(cloneLayer.style.pixelTop < orgnY)
{
stepY = 1;
}
if(cloneLayer.style.pixelLeft == orgnX)
{
stepX = 0;
}
if(cloneLayer.style.pixelTop == orgnY)
{
stepY = 0;
}
if(cloneLayer.style.pixelLeft == orgnX && cloneLayer.style.pixelTop == orgnY)
{
EndMove();
}
}
//to the design
else
{
EndMove();
}
}
//stop and then back to the state ()carton
function EndMove()
{
sourceLayer.style.pixelLeft = orgnX;
sourceLayer.style.pixelTop = orgnY;
cloneLayer.style.pixelLeft = orgnX;
cloneLayer.style.pixelTop = orgnY;
cloneLayer.className = "docked";
clearInterval(back);
}
//Main function of this demo
function startDraging(inAim,inSource,inClone,initAimX,initAimY,initOrgnX,initOrgnY)
{
getLayer(inAim,inSource,inClone)
initDrag(initAimX,initAimY,initOrgnX,initOrgnY);
sourceLayer.onmousedown = BeforeDrag;
document.onmousemove = OnDrag; //if we use cloneLayer,then the content will be draged ,and well a bug
cloneLayer.onmouseup = EndDrag;
}
//transfer
startDraging("aim","sourceLayer","cloneLayer",600,500,50,50);
//-->
</script>
</body>
</html>
posted @
2007-01-29 14:42 wqwqwqwqwq 閱讀(633) |
評論 (0) |
編輯 收藏
As beginning,still don't know how to begin this post~but really should begin to write something about tech.Sometimes some one like to talk that A good bazoo can
make a good bussiness card~,may be so,may be not,I really want to throw it away,but cannot,just can to learn and write ...off topics...
From now I propose to write a route about js tech with my learning steps.Ajax is a good thing maybe the world just like it some good but really short. Js is called for the full JavaScriptlanguage,it's welcomed because it can be run at the piont of client and also effective.when we talk about java object orientation is the focus piont which attracts our attention.we can define a class and then a function.But to JS ,when we create a function and make a instance of this function,we just regard it as a class,so we may say a newable function was a class.The class may have its own attributes or methods,but now we can use the sign["~"]to quote it(refer to).
Now I give a simple example about this

Of course,u can express with arr.push("Eric").After this,I want to say,we can insert,update & delete attributes or methods when we need.As following
user.name="Eric";
user.age="21";
user.sex="male";
//we insert 3 Atts/
user.show=function(){
alert("Name is"+this.name);
}
//we insert 1 Mes/
delete is very very easy,a old story.. we use undefined
user.name=undefined;
user.show=undefined;
//....
This is js today's post,easiness goes,.~ ..
posted @
2007-01-27 23:17 wqwqwqwqwq 閱讀(635) |
評論 (0) |
編輯 收藏
java的i/o恐怕是java體系里最復雜的內容之一了,有時候這個之一可以去了。不像c語言一個fopen()就可以解決一大堆問題。到了java
呢,又是stream又是reader,讀一個文件的方式不下十種,造成很多人因此而放棄了java,當初剛學java的時候班里很多人都對java有很
高的熱情,可到了現在,不知道還有多少人堅持下來了。具有諷刺意味的事java
i/o的設計者的初衷是讓i/o變得簡單一點,哪知道若干年后,弄出這么一大攤子來。現在又加了一個nio----就是newio,不知道日后還會弄出什
么東西來。
用i/o可以解決文件,網絡通訊等幾乎所io問題。功能強大,唯一的缺點就是復雜。但仔細一分析,還是有門路可走的。整個io體系主要分為兩大門派。一派
為流類也就是用于字節的InputStream和OutputStream,另一派為用于字符的Reader和Writer派(簡稱rw派)。認清了這兩
派就知道*Stream的東東全都是流派的,不是繼承而來就是通過實現接口而來。如此,*Reader,*Writer那就是出身于rw派了。還有一個
File派來處理文件創建,刪除,修改,屬性問題。對文件的內容進行操作不是他的工作。搞清楚這兩大派一小派那么解決io指日可待。
那么說了這么多怎么創建文件呢?舉例說明(主函數就不寫了)
File newfile = new File("text.txt");
newfile.createNewFile();
這樣就在同一個文件夾下創建了一個名為text.txt的文本文件,那枚怎么刪除呢?
File newfile = new File("text.txt");
newfile.delete();
這樣就把這個文件刪除了。是不是很簡單呢。那么怎么把文件寫入這個文本文件呢?
File writetext = new File("text.txt");
[readtext.createNewFile();]//可有可無,因為文件不存在的話,會自動創建
FileWriter fw = new FileWriter(writetext);//就像創建打印機
PrintWriter pw = new PrintWriter(fw);//這個呢打印針頭了。
pw.println("this is a new file for
read");//這個是說,把這些東西給我寫進去吧
fw.close();//然后關閉打印機
有點復雜,當懂了以后,也就感覺不到什么了,那么如何讀取這個文本文件呢?
File readtext = new File("text.txt");
FileReader fr = new FileReaer(readtext);//創建一個掃描儀
BufferedReader br = new
BufferedReader(fr);//這是掃描儀內的緩存
String content =
br.readLine(); //讀一行文本
用熟練了之后可以這么寫:
BufferedReader in = new BufferedReader(new
FileReader("text.txt");
String content="";
while((content=in.readLine())!=null){
System.out.println(content);//讀一行輸出一行
}
posted @
2006-12-04 13:27 wqwqwqwqwq 閱讀(367) |
評論 (0) |
編輯 收藏
jar2exe是國產軟件,看來這方面的需求還是很旺盛的。點擊
這里下載。
過程極為簡單,第一步
第二步,選擇應用程序類型
第三步,輸入啟動類。輸入帶有 main 方法的類名
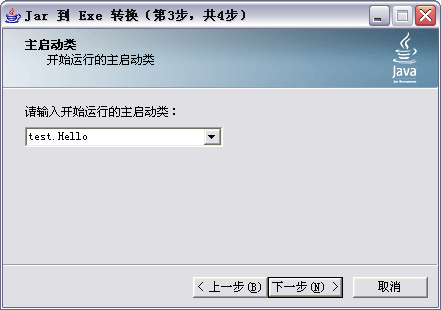
第四步,輸入要生成的 Exe 文件名:
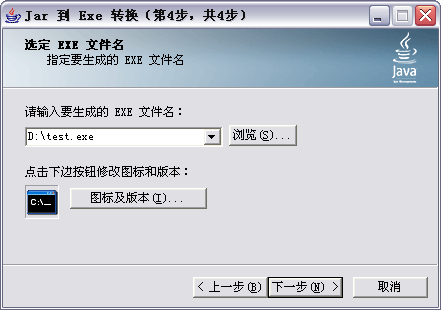
posted @
2006-12-04 13:18 wqwqwqwqwq 閱讀(1586) |
評論 (0) |
編輯 收藏